linux环境 使用命名管道作为两个进程间传输消息实例代码
时间: 2023-09-16 17:13:40 浏览: 79
下面是一个简单的使用命名管道作为两个进程间传输消息的示例代码:
发送方代码:
```c
#include <fcntl.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/stat.h>
#include <unistd.h>
#define FIFO_FILE "/tmp/myfifo"
int main()
{
int fd;
char *message = "Hello World\n";
mode_t mode = S_IRUSR | S_IWUSR | S_IRGRP | S_IROTH;
// 创建 FIFO 管道
mkfifo(FIFO_FILE, mode);
// 打开 FIFO 管道并向其中写入消息
fd = open(FIFO_FILE, O_WRONLY);
write(fd, message, strlen(message));
close(fd);
// 删除 FIFO 管道
unlink(FIFO_FILE);
return 0;
}
```
接收方代码:
```c
#include <fcntl.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/stat.h>
#include <unistd.h>
#define FIFO_FILE "/tmp/myfifo"
int main()
{
int fd;
char message[100];
mode_t mode = S_IRUSR | S_IWUSR | S_IRGRP | S_IROTH;
// 创建 FIFO 管道
mkfifo(FIFO_FILE, mode);
// 打开 FIFO 管道并从其中读取消息
fd = open(FIFO_FILE, O_RDONLY);
read(fd, message, sizeof(message));
printf("Received message: %s", message);
close(fd);
// 删除 FIFO 管道
unlink(FIFO_FILE);
return 0;
}
```
这里的示例代码中,发送方使用 `open` 和 `write` 函数向 FIFO 管道中写入消息,接收方使用 `open` 和 `read` 函数从 FIFO 管道中读取消息。需要注意的是,发送方和接收方都需要先创建 FIFO 管道,然后再进行消息的发送和接收。在完成消息传输后,需要将 FIFO 管道删除,以释放相关资源。
阅读全文
相关推荐

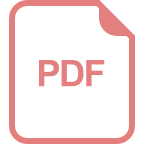
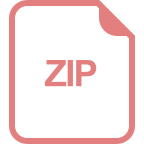
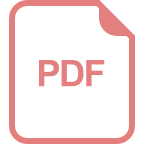
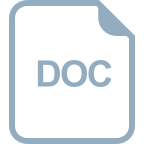
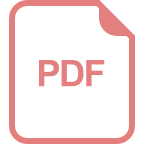
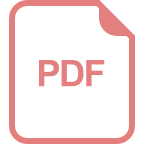
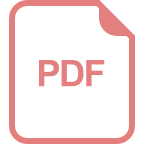
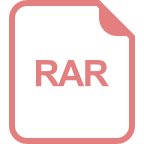
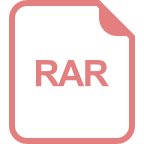
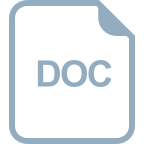
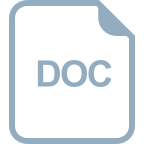
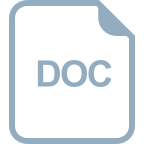
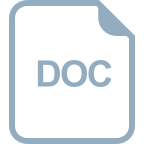
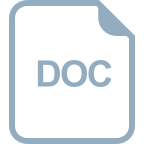