实现一个通讯录程序。格式为,姓名,单位,电话,住址,宅电,要求建立Person类,然后使用文件流保存和显示
时间: 2023-06-15 09:04:31 浏览: 76
好的,下面是通讯录程序的实现过程:
首先,需要定义一个Person类,其中包含姓名、单位、电话、住址、宅电等信息。代码如下:
```cpp
#include <iostream>
#include <string>
#include <fstream>
using namespace std;
class Person {
public:
string name; // 姓名
string company; // 单位
string phone; // 电话
string address; // 住址
string home; // 宅电
// 构造函数
Person(string n, string c, string p, string a, string h) {
name = n;
company = c;
phone = p;
address = a;
home = h;
}
// 默认构造函数
Person() {}
// 显示个人信息
void display() {
cout << "姓名:" << name << endl;
cout << "单位:" << company << endl;
cout << "电话:" << phone << endl;
cout << "住址:" << address << endl;
cout << "宅电:" << home << endl;
}
};
```
接下来,需要实现文件流的读写操作,将通讯录数据保存到文件中并读取出来。代码如下:
```cpp
// 将通讯录保存到文件
void saveToTxt(Person* p, int count) {
ofstream fout("contacts.txt");
for (int i = 0; i < count; ++i) {
fout << p[i].name << "," << p[i].company << "," << p[i].phone << ","
<< p[i].address << "," << p[i].home << endl;
}
fout.close();
}
// 从文件中读取通讯录
void readFromTxt(Person* p, int& count) {
ifstream fin("contacts.txt");
if (!fin.is_open()) {
cout << "文件不存在!" << endl;
return;
}
string line;
while (getline(fin, line)) {
string name, company, phone, address, home;
int pos1 = line.find(",");
int pos2 = line.find(",", pos1 + 1);
int pos3 = line.find(",", pos2 + 1);
int pos4 = line.find(",", pos3 + 1);
name = line.substr(0, pos1);
company = line.substr(pos1 + 1, pos2 - pos1 - 1);
phone = line.substr(pos2 + 1, pos3 - pos2 - 1);
address = line.substr(pos3 + 1, pos4 - pos3 - 1);
home = line.substr(pos4 + 1);
p[count] = Person(name, company, phone, address, home);
++count;
}
fin.close();
}
```
最后,实现主函数,往通讯录中添加联系人,并显示通讯录中所有联系人的信息。代码如下:
```cpp
int main() {
Person p[100];
int count = 0;
// 添加联系人
p[count++] = Person("张三", "ABC公司", "13800138000", "北京市海淀区", "010-12345678");
p[count++] = Person("李四", "XYZ公司", "13900139000", "北京市朝阳区", "010-87654321");
// 将通讯录保存到文件
saveToTxt(p, count);
// 从文件中读取通讯录
readFromTxt(p, count);
// 显示通讯录中所有联系人的信息
for (int i = 0; i < count; ++i) {
p[i].display();
cout << endl;
}
return 0;
}
```
运行程序后,通讯录中所有联系人的信息将会被显示出来。
相关推荐
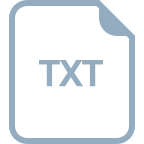
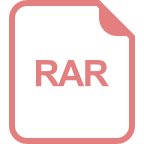














