vue base64转码
时间: 2023-10-01 07:03:09 浏览: 155
在Vue中进行Base64编码和解码可以使用JavaScript自带的btoa和atob函数。btoa函数可以将字符串编码为Base64格式,而atob函数可以将Base64格式的字符串解码为原始字符串。
以下是一个示例代码,将一个字符串进行Base64编码并输出到控制台:
```
let str = 'Hello World!';
let encoded = btoa(str);
console.log(encoded);
```
输出结果为:`"SGVsbG8gV29ybGQh"`,这就是字符串"Hello World!"的Base64编码结果。
以下是一个示例代码,将一个Base64格式的字符串进行解码并输出到控制台:
```
let encoded = 'SGVsbG8gV29ybGQh';
let decoded = atob(encoded);
console.log(decoded);
```
输出结果为:"Hello World!",这就是Base64编码为"SGVsbG8gV29ybGQh"的原始字符串。
相关问题
vue如何设置base64转码后的内容为utf-8
在Vue中,可以使用atob()函数将Base64编码解码为原始字符串,然后使用decodeURIComponent()函数将已编码URI组件解码, 从而将UTF-8编码的字符串转换成JavaScript字符串。
例如:
```
let base64str = "VGhpcyBpcyBhIHRlc3Qh"; //假设这是一个Base64编码的字符串
let decodedStr = decodeURIComponent(atob(base64str).split('').map(function(c) {
return '%' + ('00' + c.charCodeAt(0).toString(16)).slice(-2);
}).join(''));
console.log(decodedStr); //输出 "This is a test!"
```
在上面的代码中,我们首先使用atob()函数将Base64编码解码为原始字符串,然后使用split()函数将字符串转换为字符数组,并使用map()函数对每个字符进行操作,最后使用join()函数将字符数组转换回字符串。最终,我们得到了一个解码后的UTF-8字符串。
vue中c盘图片路径转base64
根据提供的引用内容,可以使用require将变量中保存的图片地址转码为base64。具体步骤如下:
1. 将需要转码的图片放到项目文件夹的static文件夹下,例如将C盘中的图片复制到项目文件夹的static文件夹下。
2. 在Vue组件中,使用require引入图片,并将其赋值给一个变量,例如:
```
<template>
<div>
<img :src="imgUrl" alt="图片">
</div>
</template>
<script>
export default {
data() {
return {
imgUrl: require('@/static/image.jpg')
}
}
}
</script>
```
在上述代码中,@符号表示src目录,因此@/static/image.jpg表示项目文件夹下的static文件夹中的image.jpg图片。
3. 在浏览器中打开该Vue组件,图片将会以base64的形式显示出来。
阅读全文
相关推荐
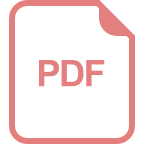
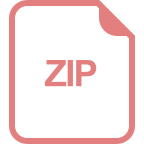
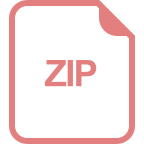
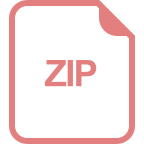
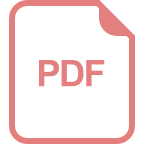
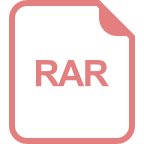
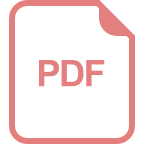
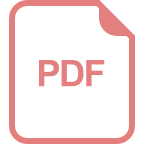
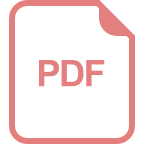
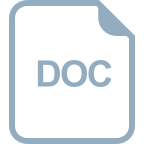
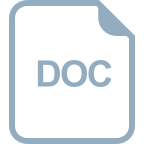
