Node.js如何查询
时间: 2024-11-06 19:05:37 浏览: 13
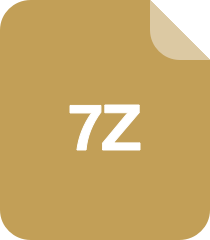
node.js14.18.3版本下载
在Node.js中,查询通常是通过各种数据库驱动程序(例如MongoDB、MySQL、PostgreSQL等)或API接口来实现的。以下是针对不同数据库查询的基本步骤:
### 对于关系型数据库(如MySQL、PostgreSQL):
1. 安装相应的数据库模块,如`mysql2`或`pg`.
```bash
npm install mysql2 pg
```
2. 使用连接池创建数据库连接:
```javascript
const mysql = require('mysql2/promise');
const pool = mysql.createPool({
host: 'localhost',
user: 'username',
password: 'password',
database: 'database_name'
});
```
3. 查询数据:
```javascript
pool.query('SELECT * FROM table_name', (err, results) => {
if (err) throw err;
console.log(results);
});
```
### 对于NoSQL数据库(如MongoDB):
1. 安装`mongodb`模块:
```bash
npm install mongodb
```
2. 连接数据库:
```javascript
const MongoClient = require('mongodb').MongoClient;
const uri = "mongodb+srv://<username>:<password>@cluster0.mongodb.net/test?retryWrites=true&w=majority";
MongoClient.connect(uri, { useNewUrlParser: true, useUnifiedTopology: true }, (err, client) => {
if (err) return console.error(err);
const db = client.db('test');
// 查询数据
db.collection('collection_name').find().toArray((err, docs) => {
if (err) throw err;
console.log(docs);
client.close(); // 关闭连接
});
});
```
### API查询(如HTTP请求):
使用`axios`或`request`等库发起HTTP GET请求:
```javascript
const axios = require('axios');
axios.get('https://api.example.com/data')
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error(error);
});
```
对于更复杂的查询(分页、筛选、聚合等),需要查看对应数据库的文档学习其API或使用ORM工具,如Sequelize、Mongoose等。
阅读全文
相关推荐
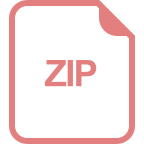
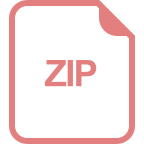
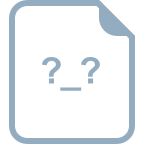
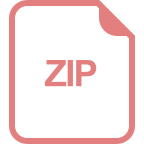
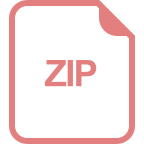
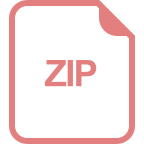
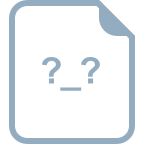
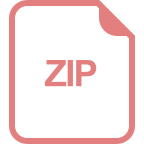
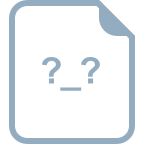
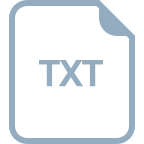
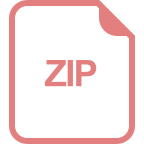
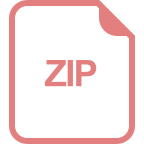
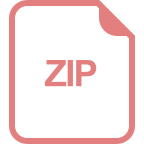
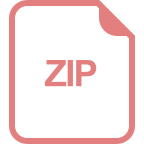
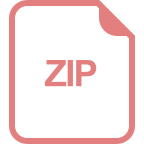