C语言的词法分析器代码
时间: 2023-05-29 20:06:48 浏览: 58
这是一个简单的C语言词法分析器代码,可以识别C语言中的关键字、标识符、常量和运算符。
```c
#include <stdio.h>
#include <ctype.h>
#include <string.h>
#define MAX_TOKEN_LENGTH 100
typedef enum {
KEYWORD,
IDENTIFIER,
NUMBER,
OPERATOR
} TokenType;
typedef struct {
TokenType type;
char value[MAX_TOKEN_LENGTH];
} Token;
Token getNextToken(FILE *fp);
int isKeyword(char *token);
int isOperator(char ch);
int main() {
FILE *fp;
Token token;
fp = fopen("input.c", "r");
if(fp == NULL) {
printf("Error opening file.\n");
return 1;
}
while((token = getNextToken(fp)).type != EOF) {
printf("%s\n", token.value);
}
fclose(fp);
return 0;
}
Token getNextToken(FILE *fp) {
Token token;
char ch;
int i = 0;
token.type = EOF;
memset(token.value, 0, MAX_TOKEN_LENGTH);
while((ch = fgetc(fp)) != EOF) {
if(isspace(ch)) {
continue;
}
if(isalpha(ch)) {
token.type = IDENTIFIER;
token.value[i++] = ch;
while(isalnum(ch = fgetc(fp))) {
token.value[i++] = ch;
}
ungetc(ch, fp);
if(isKeyword(token.value)) {
token.type = KEYWORD;
}
break;
}
if(isdigit(ch)) {
token.type = NUMBER;
token.value[i++] = ch;
while(isdigit(ch = fgetc(fp))) {
token.value[i++] = ch;
}
ungetc(ch, fp);
break;
}
if(isOperator(ch)) {
token.type = OPERATOR;
token.value[i++] = ch;
if((ch == '>' || ch == '<') && (ch = fgetc(fp)) == '=') {
token.value[i++] = ch;
} else {
ungetc(ch, fp);
}
break;
}
}
return token;
}
int isKeyword(char *token) {
char *keywords[] = {"auto", "break", "case", "char", "const", "continue",
"default", "do", "double", "else", "enum", "extern",
"float", "for", "goto", "if", "int", "long", "register",
"return", "short", "signed", "sizeof", "static",
"struct", "switch", "typedef", "union", "unsigned",
"void", "volatile", "while"};
int i, numKeywords = sizeof(keywords) / sizeof(keywords[0]);
for(i = 0; i < numKeywords; i++) {
if(strcmp(token, keywords[i]) == 0) {
return 1;
}
}
return 0;
}
int isOperator(char ch) {
char operators[] = "+-*/%=&|<>^!~?";
int i, numOperators = sizeof(operators) / sizeof(operators[0]);
for(i = 0; i < numOperators; i++) {
if(ch == operators[i]) {
return 1;
}
}
return 0;
}
```
该代码通过`getNextToken`函数逐个读取输入文件中的字符,并根据字符类型生成相应的Token。`isKeyword`和`isOperator`函数用于判断识别出的Token是否为关键字和运算符。程序将Token的类型和值保存在Token结构体中,并在主函数中输出Token的值。
该代码仅仅是一个简单的词法分析器,无法处理C语言中的复杂语法结构,例如函数调用、数组等。对于更复杂的语法分析,需要使用更复杂的算法和数据结构,例如递归下降分析器、自动机等。
相关推荐
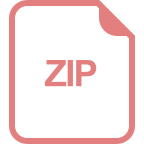
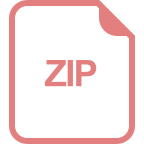
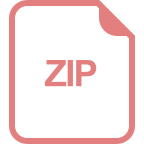














