编写一个Python程序,可以打印出H3C交换机的版本信息
时间: 2023-08-31 18:24:50 浏览: 130
### 回答1:
可以使用Python脚本获取H3C交换机的版本信息,脚本如下:import paramiko# 创建 SSH 对象
ssh = paramiko.SSHClient()# 允许连接不在known_hosts文件中的主机
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())# 连接服务器
ssh.connect(hostname='H3C Switch IP', port=22, username='username', password='password')# 执行命令
stdin, stdout, stderr = ssh.exec_command('display version')# 获取命令结果
result = stdout.read().decode('utf-8')# 关闭连接
ssh.close()print(result)
### 回答2:
编写一个Python程序,可以打印出H3C交换机的版本信息。我们可以使用Paramiko库来连接到交换机,并通过SSH协议发送命令来获取版本信息。
首先,我们需要使用pip安装Paramiko库。打开终端并运行以下命令:
```
pip install paramiko
```
接下来,我们可以编写Python程序。以下是一个示例程序:
```python
import paramiko
def get_switch_version(ip, username, password):
# 创建SSH client
ssh = paramiko.SSHClient()
# 添加SSH密钥
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
try:
# 连接到交换机
ssh.connect(ip, username=username, password=password)
# 发送命令获取版本信息
stdin, stdout, stderr = ssh.exec_command("display version")
# 读取输出并打印版本信息
output = stdout.read().decode("utf-8")
print(output)
# 关闭SSH连接
ssh.close()
except paramiko.AuthenticationException:
print("Authentication failed")
except paramiko.SSHException as e:
print("SSH connection failed:", str(e))
# 改为正确的交换机IP地址、用户名和密码
ip = "交换机IP地址"
username = "用户名"
password = "密码"
get_switch_version(ip, username, password)
```
请将代码中的ip、username和password改为实际的交换机IP地址、用户名和密码。
运行程序后,它将连接到交换机并发送"display version"命令。然后,它将打印出交换机的版本信息。
### 回答3:
要编写一个Python程序来打印H3C交换机的版本信息,可以使用SNMP协议来获取交换机的系统描述信息。
首先,我们需要安装PySNMP库,这是一个用于实现SNMP协议的Python库。可以通过以下命令来安装:
```
pip install pysnmp
```
然后,我们可以编写一个Python程序,使用PySNMP库来获取交换机的版本信息。以下是一个示例程序:
```python
from pysnmp.hlapi import *
def get_h3c_version(ip, community):
g = getCmd(SnmpEngine(),
CommunityData(community),
UdpTransportTarget((ip, 161)),
ContextData(),
ObjectType(ObjectIdentity('SNMPv2-MIB', 'sysDescr', 0)))
error_indication, error_status, error_index, var_binds = next(g)
if error_indication:
print('Error: %s' % error_indication)
elif error_status:
print('Error: %s at %s' % (error_status.prettyPrint(), var_binds[int(error_index)-1] if error_index else '?'))
else:
for var_bind in var_binds:
print('H3C Version: %s' % var_bind[1])
# 示例用法
get_h3c_version('交换机IP地址', 'SNMP团体字符串')
```
在示例程序中,`get_h3c_version`函数接受交换机的IP地址和SNMP团体字符串作为参数,并使用SNMP协议获取交换机的系统描述信息。然后,程序会打印出H3C交换机的版本信息。
请根据实际情况替换示例程序中的IP地址和SNMP团体字符串。确保你的计算机和交换机在同一个网络中,并且能够通过SNMP协议进行通信。
注意:在使用SNMP协议时,请确保遵守相关的法律法规和网络安全政策。
阅读全文
相关推荐
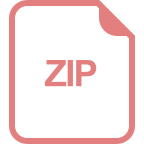
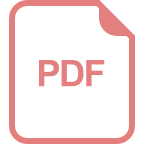

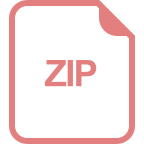
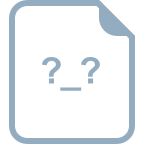
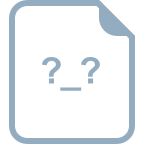
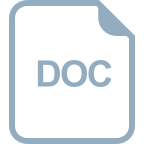
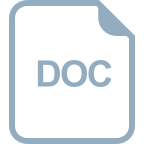
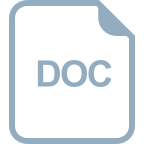
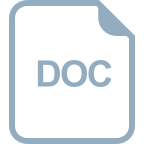
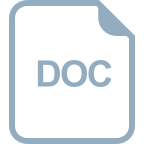
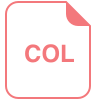
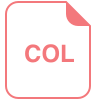




