写一个飞机订票系统可以具有录入,查询,订票,退票等功能
时间: 2023-08-04 14:05:23 浏览: 50
好的,以下是一个简单的飞机订票系统的Python代码示例:
```
class Flight:
def __init__(self, flight_number, departure_date, departure_time, arrival_time, origin, destination, seats_available):
self.flight_number = flight_number
self.departure_date = departure_date
self.departure_time = departure_time
self.arrival_time = arrival_time
self.origin = origin
self.destination = destination
self.seats_available = seats_available
class Booking:
def __init__(self, flight, passenger_name, seat_number):
self.flight = flight
self.passenger_name = passenger_name
self.seat_number = seat_number
class FlightBookingSystem:
def __init__(self):
self.flights = []
self.bookings = []
def add_flight(self, flight):
self.flights.append(flight)
def search_flights(self, origin, destination, departure_date):
matching_flights = []
for flight in self.flights:
if flight.origin == origin and flight.destination == destination and flight.departure_date == departure_date:
matching_flights.append(flight)
return matching_flights
def book_flight(self, flight_number, passenger_name, seat_number):
for flight in self.flights:
if flight.flight_number == flight_number:
if flight.seats_available > 0:
booking = Booking(flight, passenger_name, seat_number)
self.bookings.append(booking)
flight.seats_available -= 1
return booking
else:
return None
def cancel_booking(self, booking):
booking.flight.seats_available += 1
self.bookings.remove(booking)
# 示例用法
flight_system = FlightBookingSystem()
# 添加航班
flight_system.add_flight(Flight("AC101", "2022-01-01", "10:00", "12:00", "Toronto", "Vancouver", 10))
flight_system.add_flight(Flight("AC102", "2022-01-01", "14:00", "16:00", "Vancouver", "Toronto", 20))
# 搜索航班
matching_flights = flight_system.search_flights("Toronto", "Vancouver", "2022-01-01")
for flight in matching_flights:
print(f"Flight {flight.flight_number} from {flight.origin} to {flight.destination} on {flight.departure_date} at {flight.departure_time}")
# 预订航班
booking = flight_system.book_flight("AC101", "Alice", "A1")
if booking:
print(f"Booked flight {booking.flight.flight_number} for {booking.passenger_name} on seat {booking.seat_number}")
else:
print("No available seats for flight AC101")
# 取消预订
flight_system.cancel_booking(booking)
```
该系统使用Flight类表示航班,Booking类表示预订。FlightBookingSystem类则用于管理航班和预订,并提供录入、查询、订票和退票功能。在示例用法中,我们添加了两个航班,搜索了从Toronto到Vancouver的航班,预订了AC101航班并取消了预订。
相关推荐
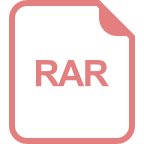
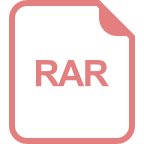
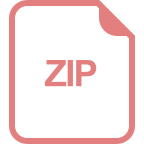














