File folder = new File("D:/folderName");if (!folder.exists()) { folder.mkdir();}怎么连起来File file = new File("D:/folderName/2.io");if (!file.exists()) { file.createNewFile();}
时间: 2024-03-15 10:45:47 浏览: 11
可以将创建文件和创建文件夹的代码连起来,如下所示:
```
File folder = new File("D:/folderName");
if (!folder.exists()) {
folder.mkdir();
}
File file = new File("D:/folderName/2.io");
if (!file.exists()) {
file.createNewFile();
}
```
这样,如果文件夹不存在,会先创建文件夹,然后再创建文件。如果文件夹已经存在,直接创建文件。
相关问题
在d盘下创建文件夹,在文件夹之下创建一个文件,名字首字母缩写或全称英文作为文件名2.io流里面的输出流往新建的文件里写内容 3.io流里面的输入流读取新建的文件里写内容 4.便用stringbuffer来对内容进行截取(5-10)
好的,让我来回答你的问题:
1. 在D盘下创建文件夹,可以使用以下代码:
```java
File folder = new File("D:/folderName");
if (!folder.exists()) {
folder.mkdir();
}
```
2. 在文件夹之下创建一个文件,可以使用以下代码:
```java
File file = new File("D:/folderName/2.io");
if (!file.exists()) {
file.createNewFile();
}
```
3. 使用输出流往新建的文件里写内容,可以使用以下代码:
```java
FileOutputStream fos = new FileOutputStream(file);
OutputStreamWriter osw = new OutputStreamWriter(fos, "UTF-8");
osw.write("这是写入文件的内容");
osw.flush();
osw.close();
```
4. 使用输入流读取新建的文件里的内容,可以使用以下代码:
```java
FileInputStream fis = new FileInputStream(file);
InputStreamReader isr = new InputStreamReader(fis, "UTF-8");
char[] buffer = new char[1024];
int len;
StringBuffer sb = new StringBuffer();
while ((len = isr.read(buffer)) != -1) {
sb.append(buffer, 0, len);
}
isr.close();
String content = sb.toString();
```
5. 使用StringBuffer来对内容进行截取(5-10),可以使用以下代码:
```java
String subContent = sb.substring(5, 11);
```
希望我的回答能够帮助你解决问题,如果还有其他问题,请随时提出。
在d盘下创建文件夹,在文件夹之下创建一个文件,名字首字母缩写或全称英文作为文件名io流里面的输出流往新建的文件里写内容io流里面的输入流读取新建的文件里写内容 便用stringbuffer来对内容进行截取(5-10)
好的,让我来回答你的问题:
1. 在D盘下创建文件夹,可以使用以下代码:
```java
File folder = new File("D:/folderName");
if (!folder.exists()) {
folder.mkdir();
}
```
2. 在文件夹之下创建一个文件,可以使用以下代码:
```java
File file = new File("D:/folderName/io.txt");
if (!file.exists()) {
file.createNewFile();
}
```
3. 使用输出流往新建的文件里写内容,可以使用以下代码:
```java
FileOutputStream fos = new FileOutputStream(file);
OutputStreamWriter osw = new OutputStreamWriter(fos, "UTF-8");
osw.write("这是写入文件的内容");
osw.flush();
osw.close();
```
4. 使用输入流读取新建的文件里的内容,可以使用以下代码:
```java
FileInputStream fis = new FileInputStream(file);
InputStreamReader isr = new InputStreamReader(fis, "UTF-8");
char[] buffer = new char[1024];
int len;
StringBuffer sb = new StringBuffer();
while ((len = isr.read(buffer)) != -1) {
sb.append(buffer, 0, len);
}
isr.close();
String content = sb.toString();
```
5. 使用StringBuffer来对内容进行截取(5-10),可以使用以下代码:
```java
String subContent = sb.substring(5, 11);
```
希望我的回答能够帮助你解决问题,如果还有其他问题,请随时提出。
相关推荐
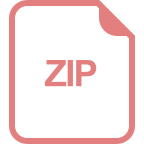
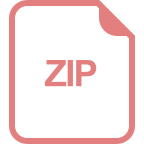
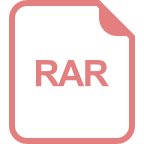











