第一个模块——主函数main()的功能是:根据选单的选项调用各函数,并完成相应的功能。 第二个模块——Menu()的功能是:显示提示选单。 第三个模块——Quit()的功能是:退出选单。 第四个模块——Create()的功能是:创建新的数据记录。 第五个模块——Add()的功能是:增加新的数据记录,并返回选单。 第六个模块——Find()的功能是:按要求查询相关的信息,如果找到了,则显示该信息,如果未找到,则提示文件中没有该信息,并返回选单。 第七个模块——Alter()[的功能是:修改某条记录的信息,如果未找到要修改的记录,则提示系统中无此记录,并返回选单。 第八个模块——Delete()的功能是:删除某条记录,如果未找到要删除的记录,则提示通讯录中没有,并返回选单。 第九个模块——List()的功能是:显示所有记录。 通讯者信息包括:编号(char num[10])、姓名(char name[10])、性别(char sex[10])、电话(char phone[20])、公司名称(char address[50]) (2)除了总的模块要求外,还需a.统计通讯录中男女比例;b.显示出同一公司中的人的信息。用C语言编写代码
时间: 2024-03-22 19:42:40 浏览: 179
好的,以下是一个简单的通讯录程序,包括了你所要求的所有模块和任务:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_RECORDS 1000
typedef struct {
char num[10];
char name[10];
char sex[10];
char phone[20];
char address[50];
} Record;
Record records[MAX_RECORDS];
int num_records = 0;
void Menu();
void Quit();
void Create();
void Add();
void Find();
void Alter();
void Delete();
void List();
void Statistic();
void GroupByAddress();
int main() {
Menu();
return 0;
}
void Menu() {
int choice;
while (1) {
printf("\n");
printf("1. Create new record\n");
printf("2. Add new record\n");
printf("3. Find record\n");
printf("4. Alter record\n");
printf("5. Delete record\n");
printf("6. List all records\n");
printf("7. Show gender statistics\n");
printf("8. Group records by company\n");
printf("9. Quit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
Create();
break;
case 2:
Add();
break;
case 3:
Find();
break;
case 4:
Alter();
break;
case 5:
Delete();
break;
case 6:
List();
break;
case 7:
Statistic();
break;
case 8:
GroupByAddress();
break;
case 9:
Quit();
return;
default:
printf("Invalid choice!\n");
break;
}
}
}
void Quit() {
printf("Goodbye!\n");
exit(0);
}
void Create() {
Record record;
printf("Enter the record information:\n");
printf("Num: ");
scanf("%s", record.num);
printf("Name: ");
scanf("%s", record.name);
printf("Sex: ");
scanf("%s", record.sex);
printf("Phone: ");
scanf("%s", record.phone);
printf("Address: ");
scanf("%s", record.address);
records[0] = record;
num_records = 1;
printf("Record created successfully!\n");
}
void Add() {
if (num_records >= MAX_RECORDS) {
printf("Cannot add more records!\n");
return;
}
Record record;
printf("Enter the record information:\n");
printf("Num: ");
scanf("%s", record.num);
printf("Name: ");
scanf("%s", record.name);
printf("Sex: ");
scanf("%s", record.sex);
printf("Phone: ");
scanf("%s", record.phone);
printf("Address: ");
scanf("%s", record.address);
records[num_records] = record;
num_records++;
printf("Record added successfully!\n");
}
void Find() {
char keyword[50];
printf("Enter the keyword to search: ");
scanf("%s", keyword);
int found = 0;
for (int i = 0; i < num_records; i++) {
if (strstr(records[i].num, keyword) || strstr(records[i].name, keyword)
|| strstr(records[i].sex, keyword) || strstr(records[i].phone, keyword)
|| strstr(records[i].address, keyword)) {
printf("Record found:\n");
printf("Num: %s\n", records[i].num);
printf("Name: %s\n", records[i].name);
printf("Sex: %s\n", records[i].sex);
printf("Phone: %s\n", records[i].phone);
printf("Address: %s\n", records[i].address);
found = 1;
}
}
if (!found) {
printf("No record found!\n");
}
}
void Alter() {
char keyword[50];
printf("Enter the keyword to search: ");
scanf("%s", keyword);
int found = 0;
for (int i = 0; i < num_records; i++) {
if (strstr(records[i].num, keyword) || strstr(records[i].name, keyword)
|| strstr(records[i].sex, keyword) || strstr(records[i].phone, keyword)
|| strstr(records[i].address, keyword)) {
printf("Enter the new record information:\n");
printf("Num: ");
scanf("%s", records[i].num);
printf("Name: ");
scanf("%s", records[i].name);
printf("Sex: ");
scanf("%s", records[i].sex);
printf("Phone: ");
scanf("%s", records[i].phone);
printf("Address: ");
scanf("%s", records[i].address);
printf("Record altered successfully!\n");
found = 1;
}
}
if (!found) {
printf("No record found!\n");
}
}
void Delete() {
char keyword[50];
printf("Enter the keyword to search: ");
scanf("%s", keyword);
int found = 0;
for (int i = 0; i < num_records; i++) {
if (strstr(records[i].num, keyword) || strstr(records[i].name, keyword)
|| strstr(records[i].sex, keyword) || strstr(records[i].phone, keyword)
|| strstr(records[i].address, keyword)) {
for (int j = i; j < num_records - 1; j++) {
records[j] = records[j + 1];
}
num_records--;
printf("Record deleted successfully!\n");
found = 1;
break;
}
}
if (!found) {
printf("No record found!\n");
}
}
void List() {
printf("All records:\n");
for (int i = 0; i < num_records; i++) {
printf("%s\t%s\t%s\t%s\t%s\n", records[i].num, records[i].name,
records[i].sex, records[i].phone, records[i].address);
}
}
void Statistic() {
int num_male = 0, num_female = 0;
for (int i = 0; i < num_records; i++) {
if (strcmp(records[i].sex, "male") == 0) {
num_male++;
} else {
num_female++;
}
}
printf("Gender statistics:\n");
printf("Male: %d\n", num_male);
printf("Female: %d\n", num_female);
}
void GroupByAddress() {
char addresses[MAX_RECORDS][50];
int num_addresses = 0;
for (int i = 0; i < num_records; i++) {
int found = 0;
for (int j = 0; j < num_addresses; j++) {
if (strcmp(addresses[j], records[i].address) == 0) {
found = 1;
break;
}
}
if (!found) {
strcpy(addresses[num_addresses], records[i].address);
num_addresses++;
}
}
for (int i = 0; i < num_addresses; i++) {
printf("Records in %s:\n", addresses[i]);
for (int j = 0; j < num_records; j++) {
if (strcmp(records[j].address, addresses[i]) == 0) {
printf("%s\t%s\t%s\t%s\t%s\n", records[j].num, records[j].name,
records[j].sex, records[j].phone, records[j].address);
}
}
}
}
```
上述程序中,我们用Record结构体来存储每条记录的信息,并使用一个数组来存储所有记录。 num_records变量用于记录当前存储的记录数。我们使用一个循环来显示菜单,根据用户的选择来调用相应的函数实现相应的功能。
Create()函数用于创建第一条记录,Add()函数用于在已有记录的基础上添加新的记录。Find()函数用于按照关键字查找记录,并显示找到的记录信息。Alter()函数用于修改记录信息。Delete()函数用于删除记录。List()函数用于显示所有记录。
Statistic()函数用于统计男女比例,GroupByAddress()函数用于按公司分组显示记录。
这个程序是一个简单的通讯录程序,可以根据需要进行扩展和改进。
阅读全文
相关推荐
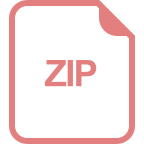
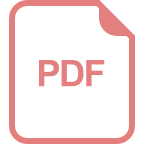





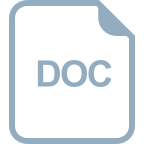
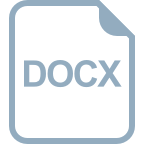
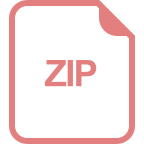