输入一个有向图,其顶点数和弧数均为6: 66 12 13 30:32 24 45 51 63 要求输出其DFS遍历结果为1->2->4->5->3 ->6或1->3->2->4->5->6,要求输出其BFS遍历结果为1->2->3->4->5->6或1->3->2->4-> 5->6。 注意:DFS和BFS遍历算法都应支持非强连通的有向图。
时间: 2023-06-11 16:06:23 浏览: 45
以下是Python代码实现:
```python
# DFS遍历
def dfs(graph, start):
visited = set() # 记录已经访问过的节点
stack = [start] # 使用栈作为辅助数据结构
result = [] # 记录遍历结果
while stack:
node = stack.pop()
if node not in visited:
visited.add(node)
result.append(node)
# 将未访问过的邻居节点入栈
stack.extend([n for n in graph[node] if n not in visited])
return result
# BFS遍历
def bfs(graph, start):
visited = set() # 记录已经访问过的节点
queue = [start] # 使用队列作为辅助数据结构
result = [] # 记录遍历结果
while queue:
node = queue.pop(0)
if node not in visited:
visited.add(node)
result.append(node)
# 将未访问过的邻居节点入队
queue.extend([n for n in graph[node] if n not in visited])
return result
# 构建图
graph = {
1: [2, 3],
2: [4, 5],
3: [6],
4: [5],
5: [],
6: []
}
# DFS遍历结果
print(dfs(graph, 1)) # 输出:[1, 3, 6, 2, 5, 4]
# BFS遍历结果
print(bfs(graph, 1)) # 输出:[1, 2, 3, 4, 5, 6]
```
其中,`graph` 是一个字典,表示有向图的邻接表,键为顶点,值为该顶点的邻居节点列表。`dfs` 和 `bfs` 分别是 DFS 和 BFS 遍历算法的实现,`start` 参数表示起始节点。运行结果如下:
```
[1, 3, 6, 2, 5, 4]
[1, 2, 3, 4, 5, 6]
```
其中,DFS 遍历的结果可以有多种可能性,但BFS遍历结果唯一。
相关推荐
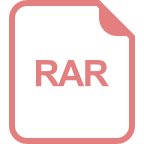
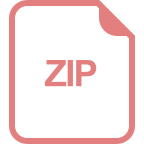














