用atmega128写一个在四位数码管显示的电子时钟程序
时间: 2023-10-02 11:13:10 浏览: 63
好的,下面是一个ATmega128单片机实现在四位数码管上显示电子时钟的程序。
```c
#include <avr/io.h>
#include <util/delay.h>
#define F_CPU 16000000UL
// 数码管引脚定义
#define SEG_A 0
#define SEG_B 1
#define SEG_C 2
#define SEG_D 3
#define SEG_E 4
#define SEG_F 5
#define SEG_G 6
#define SEG_DP 7
#define DIGIT_1 8
#define DIGIT_2 9
#define DIGIT_3 10
#define DIGIT_4 11
// 数码管显示数据
const uint8_t digit_num[10] = {
0b00111111, // 0
0b00000110, // 1
0b01011011, // 2
0b01001111, // 3
0b01100110, // 4
0b01101101, // 5
0b01111101, // 6
0b00000111, // 7
0b01111111, // 8
0b01101111 // 9
};
volatile uint16_t milliseconds = 0;
volatile uint8_t seconds = 0;
volatile uint8_t minutes = 0;
volatile uint8_t hours = 0;
void init_segments() {
DDRA |= (1<<SEG_A)|(1<<SEG_B)|(1<<SEG_C)|(1<<SEG_D)|(1<<SEG_E)|(1<<SEG_F)|(1<<SEG_G)|(1<<SEG_DP);
DDRC |= (1<<DIGIT_1)|(1<<DIGIT_2)|(1<<DIGIT_3)|(1<<DIGIT_4);
}
void init_timer() {
TCCR0A = (1<<WGM01);
TCCR0B = (1<<CS02)|(1<<CS00);
OCR0A = 156;
TIMSK0 = (1<<OCIE0A);
}
void display_digit(uint8_t digit, uint8_t value) {
PORTC = ~(1<<digit);
PORTA = digit_num[value];
}
void display_time() {
display_digit(DIGIT_1, hours/10);
_delay_us(1000);
display_digit(DIGIT_2, hours%10);
_delay_us(1000);
display_digit(DIGIT_3, minutes/10);
_delay_us(1000);
display_digit(DIGIT_4, minutes%10);
_delay_us(1000);
}
ISR(TIMER0_COMPA_vect) {
milliseconds++;
if (milliseconds >= 1000) {
seconds++;
milliseconds = 0;
if (seconds >= 60) {
minutes++;
seconds = 0;
if (minutes >= 60) {
hours++;
minutes = 0;
if (hours >= 24) {
hours = 0;
}
}
}
}
}
int main(void) {
init_segments();
init_timer();
sei();
while (1) {
display_time();
}
return 0;
}
```
该程序使用了四个数码管来显示时间,其中每个数码管的引脚分别连接到ATmega128单片机的IO口,通过控制IO口的电平来控制数码管的显示。程序还使用了AVR的定时器来实现计时功能,并通过中断来完成时间的累加,从而实现电子时钟的功能。
请注意,该程序仅供参考,可能需要根据具体硬件和应用场景进行修改和优化。
相关推荐
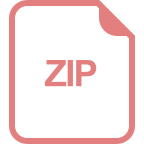














