Linux 使用C++ 写一个遍历目录下图片 图片每4张一组 每张图片文件名用下划线分割并且最后一位是图片的序号
时间: 2023-09-30 09:04:11 浏览: 71
以下是一个简单的 C++ 程序,用于遍历指定目录下的图片文件,并按照要求每4张图片为一组,每张图片的文件名用下划线分割,最后一位是图片的序号:
```cpp
#include <iostream>
#include <string>
#include <vector>
#include <algorithm>
#include <dirent.h>
using namespace std;
bool is_image_file(const string& filename) {
// 判断文件名是否以 .jpg, .jpeg, .png, .bmp 等常见图片格式为后缀
return filename.size() >= 4 && (filename.substr(filename.size() - 4) == ".jpg" ||
filename.substr(filename.size() - 5) == ".jpeg" ||
filename.substr(filename.size() - 4) == ".png" ||
filename.substr(filename.size() - 4) == ".bmp");
}
int main() {
string dir_path = "/path/to/your/directory"; // 指定目录路径
int group_size = 4; // 每组包含的图片数量
DIR* dir = opendir(dir_path.c_str());
if (dir == nullptr) {
cerr << "Failed to open directory " << dir_path << endl;
return 1;
}
vector<string> image_files;
dirent* entry;
while ((entry = readdir(dir)) != nullptr) {
string filename = entry->d_name;
if (is_image_file(filename)) {
image_files.push_back(filename);
}
}
closedir(dir);
sort(image_files.begin(), image_files.end()); // 按文件名排序
int group_num = 0;
for (int i = 0; i < image_files.size(); i += group_size) {
string group_name = "group_" + to_string(group_num);
for (int j = 0; j < group_size && i + j < image_files.size(); j++) {
string old_filename = image_files[i + j];
string new_filename = group_name + "_" + to_string(j) + old_filename.substr(old_filename.find_last_of('.'));
string old_path = dir_path + "/" + old_filename;
string new_path = dir_path + "/" + new_filename;
rename(old_path.c_str(), new_path.c_str());
}
group_num++;
}
return 0;
}
```
该程序会先读取指定目录下的所有图片文件,然后按照文件名排序,最后将每4张图片分为一组,并修改文件名以符合要求。你可以根据需要修改目录路径和每组包含的图片数量。注意,在程序中使用 `rename` 函数修改文件名时,需要保证程序具有足够的权限。
阅读全文
相关推荐
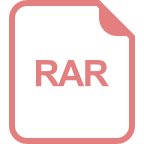
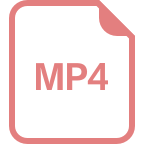
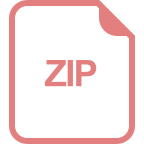
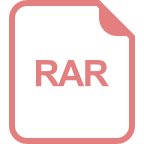
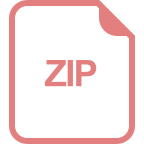
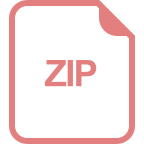
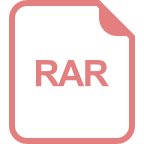
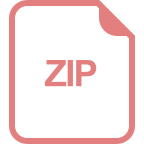
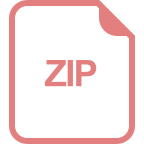