jQuery 遍历用法
时间: 2024-02-11 10:09:46 浏览: 67
jQuery提供了多种遍历DOM元素的方法,以下是一些常用的方法:
1. find()方法:查找当前元素下所有匹配选择器的子元素。
2. children()方法:查找当前元素下所有直接子元素。
3. parent()方法:查找当前元素的直接父元素。
4. parents()方法:查找当前元素的所有祖先元素,直到文档的根元素。
5. siblings()方法:查找当前元素的所有兄弟元素。
6. prev()方法:查找当前元素的上一个兄弟元素。
7. next()方法:查找当前元素的下一个兄弟元素。
8. eq()方法:选择指定索引位置的元素。
例如:
```
// 查找id为container的元素下所有class为box的子元素
$("#container").find(".box");
// 查找id为container的元素下的所有直接子元素
$("#container").children();
// 查找id为box1的元素的直接父元素
$("#box1").parent();
// 查找id为box1的元素的所有祖先元素
$("#box1").parents();
// 查找id为box1的元素的所有兄弟元素
$("#box1").siblings();
// 查找id为box1的元素的上一个兄弟元素
$("#box1").prev();
// 查找id为box1的元素的下一个兄弟元素
$("#box1").next();
// 选择第二个class为box的元素
$(".box").eq(1);
```
相关问题
jQuery 遍历表格
可以使用 jQuery 的遍历方法来遍历表格。下面是一个使用 jQuery 遍历表格的示例代码:
```html
<table>
<tr>
<th>名称</th>
<th>价格</th>
</tr>
<tr>
<td>商品1</td>
<td>$100</td>
</tr>
<tr>
<td>商品2</td>
<td>$200</td>
</tr>
</table>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script>
$(document).ready(function() {
$('table tr').each(function() {
var name = $(this).find('td:first').text();
var price = $(this).find('td:last').text();
console.log(name + ': ' + price);
});
});
</script>
```
在这个示例中,`$('table tr').each()` 方法用于遍历表格的每一行,`$(this).find('td:first')` 和 `$(this).find('td:last')` 方法用于获取当前行的第一个和最后一个单元格的内容。最后将获取到的内容输出到控制台中。
jquery遍历class
可以使用 jQuery 的 `each()` 方法来遍历指定 class 的所有元素,例如:
```javascript
$(".your-class-name").each(function() {
// 在这里处理每个匹配的元素
console.log($(this).text()); // 输出元素的文本内容
});
```
上述代码中,`$(".your-class-name")` 会选择所有拥有 `your-class-name` 类名的元素,然后使用 `each()` 方法遍历这些元素。在 `each()` 方法的回调函数中,可以通过 `$(this)` 来访问当前正在处理的元素。
阅读全文
相关推荐
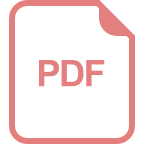
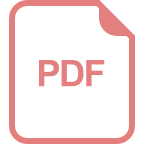
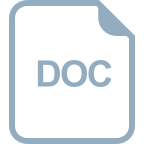
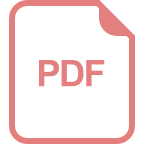
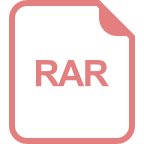
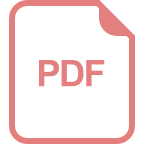
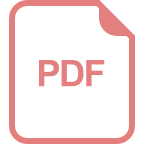
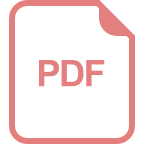
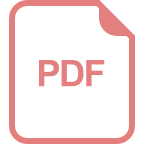
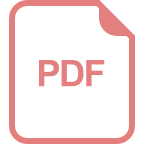
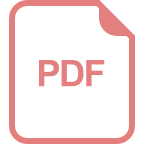
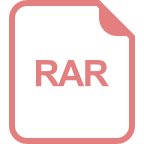
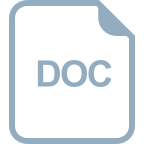
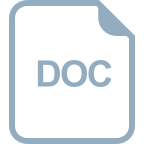
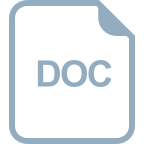