运用输入输出函数编写程序,能将华氏温度转换成摄氏温度,也可以将摄氏温度转换成华氏温度。换算公式:C=(F-32)*5/9,F=C*9/5+32其中C为摄氏温度,F为华氏温度。
时间: 2023-09-06 16:04:36 浏览: 107
### 回答1:
程序的大致思路如下: 1. 让用户输入温度值,并声明温度的单位(华氏温度或摄氏温度)。 2. 根据温度单位进行判断,如果是华氏温度,则用C=(F-32)*5/9来计算;如果是摄氏温度,则用F=C*9/5+32来计算。 3. 计算完毕后,将转换后的温度值输出。
### 回答2:
你好!要实现将华氏温度转换成摄氏温度以及将摄氏温度转换成华氏温度的功能,可以使用输入输出函数编写一个简单的程序。以下是一个示例程序:
```python
def fahrenheit_to_celsius(fahrenheit):
celsius = (fahrenheit - 32) * 5 / 9
return celsius
def celsius_to_fahrenheit(celsius):
fahrenheit = celsius * 9 / 5 + 32
return fahrenheit
def main():
temperature_type = input("请选择要转换的温度类型(输入1代表华氏温度,输入2代表摄氏温度): ")
temperature_value = float(input("请输入要转换的温度值: "))
if temperature_type == '1':
celsius = fahrenheit_to_celsius(temperature_value)
print("转换后的摄氏温度为:", celsius)
elif temperature_type == '2':
fahrenheit = celsius_to_fahrenheit(temperature_value)
print("转换后的华氏温度为:", fahrenheit)
else:
print("输入有误,请重新运行程序!")
if __name__ == "__main__":
main()
```
以上程序定义了两个函数,分别将华氏温度转换成摄氏温度和将摄氏温度转换成华氏温度。在`main()`函数中,通过用户的输入选择要转换的温度类型和输入温度值。根据温度类型的不同,调用对应的函数进行转换,并输出结果。
希望以上回答能对你有所帮助!
### 回答3:
运用输入输出函数编写程序可以实现华氏温度和摄氏温度的互相转换。首先,我们需要获取用户输入的温度值以及表示温度类型的字符。然后根据用户输入的字符选择相应的转换公式进行计算,并将计算结果输出给用户。
以下是使用Python语言编写的程序示例:
```python
def fahrenheit_to_celsius(fahrenheit):
celsius = (fahrenheit - 32) * 5 / 9
return celsius
def celsius_to_fahrenheit(celsius):
fahrenheit = celsius * 9 / 5 + 32
return fahrenheit
# 获取用户输入的温度值和温度类型
temperature = float(input("请输入温度值:"))
unit = input("请输入温度类型(C表示摄氏温度,F表示华氏温度):")
converted_temperature = 0
converted_unit = ""
if unit == "C":
converted_temperature = celsius_to_fahrenheit(temperature)
converted_unit = "华氏温度"
elif unit == "F":
converted_temperature = fahrenheit_to_celsius(temperature)
converted_unit = "摄氏温度"
else:
print("无效的温度类型!")
# 输出转换结果
print("转换结果:{}{}".format(converted_temperature, converted_unit))
```
用户输入温度值和温度类型后,程序会根据用户输入的类型进行相应的计算并输出转换结果。程序中使用了两个函数`fahrenheit_to_celsius`和`celsius_to_fahrenheit`来实现不同温度之间的转换。运行程序后,用户将获得转换后的温度值和单位类型。
相关推荐
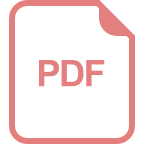
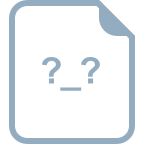














