写一个c++控制台射击游戏
时间: 2024-06-08 12:07:30 浏览: 72
好的,以下是一个简单的C++控制台射击游戏:
```cpp
#include <iostream>
#include <conio.h>
#include <Windows.h>
using namespace std;
const int WIDTH = 50; // 游戏窗口宽度
const int HEIGHT = 20; // 游戏窗口高度
int playerX = WIDTH / 2; // 玩家初始位置
int playerY = HEIGHT - 2;
int bulletX = -1; // 子弹位置
int bulletY = -1;
int enemyX = rand() % (WIDTH - 2) + 1; // 敌人初始位置
int enemyY = 1;
int score = 0; // 分数
void draw() // 绘制游戏画面
{
system("cls"); // 清屏
// 绘制游戏窗口边框
for (int i = 0; i < WIDTH + 2; i++)
cout << "#";
cout << endl;
// 绘制游戏内容
for (int i = 0; i < HEIGHT; i++) {
for (int j = 0; j < WIDTH; j++) {
if (j == 0 || j == WIDTH - 1)
cout << "#";
else if (i == playerY && j == playerX)
cout << "A"; // 绘制玩家
else if (i == enemyY && j == enemyX)
cout << "E"; // 绘制敌人
else if (i == bulletY && j == bulletX)
cout << "*"; // 绘制子弹
else
cout << " ";
}
cout << endl;
}
// 绘制分数
cout << "Score: " << score << endl;
// 绘制游戏窗口边框
for (int i = 0; i < WIDTH + 2; i++)
cout << "#";
cout << endl;
}
void update() // 更新游戏状态
{
// 更新子弹位置
if (bulletY >= 0) {
bulletY--;
if (bulletY == enemyY && bulletX == enemyX) { // 判断是否命中敌人
score++;
enemyX = rand() % (WIDTH - 2) + 1;
enemyY = 1;
bulletX = -1;
bulletY = -1;
}
else if (bulletY == 0) { // 子弹飞出屏幕
bulletX = -1;
bulletY = -1;
}
}
// 更新敌人位置
enemyY++;
if (enemyY >= HEIGHT - 1) { // 敌人到达底部
enemyX = rand() % (WIDTH - 2) + 1;
enemyY = 1;
score--;
}
// 检测玩家输入
if (_kbhit()) {
char c = _getch();
if (c == 'a' && playerX > 1)
playerX--;
if (c == 'd' && playerX < WIDTH - 2)
playerX++;
if (c == ' ')
bulletX = playerX;
bulletY = playerY - 1;
}
}
int main()
{
while (true) {
draw();
update();
Sleep(50); // 休眠一段时间,控制游戏速度
}
return 0;
}
```
这是一个非常简单的示例,只有一个敌人和一个子弹,但你可以根据需要添加更多的元素来增强游戏的复杂性和乐趣。
阅读全文
相关推荐
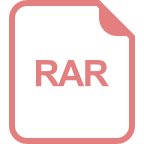
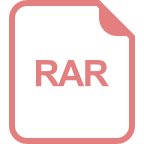
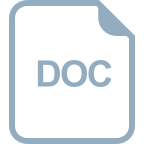
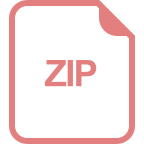
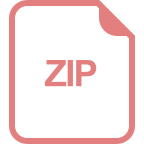
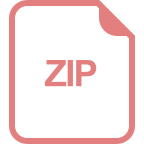
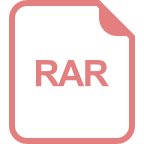
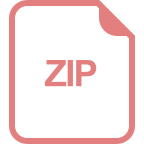
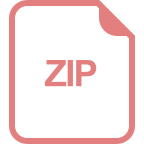
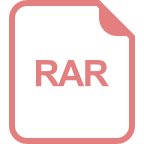
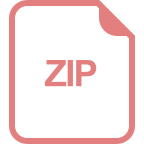
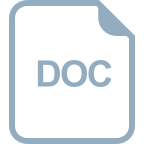
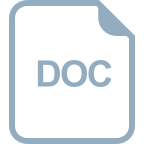

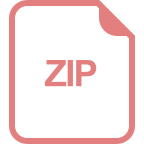
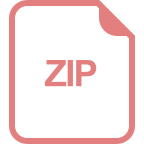
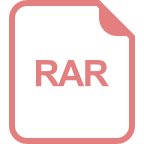
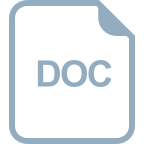