代码改进,用printf代替cout。#include <iostream> #include <queue> #include <string> using namespace std; struct Student { string name; int number; int score; }; struct TreeNode { Student data; TreeNode* left; TreeNode* right; TreeNode(Student s) : data(s), left(nullptr), right(nullptr) {} }; void inOrder(TreeNode* root) { if (!root) return; inOrder(root->left); cout << root->data.name << " " << root->data.number << " " << root->data.score << endl; inOrder(root->right); } TreeNode* insert(TreeNode* root, Student s) { if (!root) return new TreeNode(s); if (s.number < root->data.number) { root->left = insert(root->left, s); } else { root->right = insert(root->right, s); } return root; } TreeNode* search(TreeNode* root, string name) { if (!root) return nullptr; if (name == root->data.name) { return root; } else if (name < root->data.name) { return search(root->left, name); } else { return search(root->right, name); } } void print(TreeNode* root) { if (!root) return; if (root->data.score <= 75) { cout << root->data.name << " " << root->data.number << " " << root->data.score << endl; } print(root->left); print(root->right); } int main() { Student students[] = { {"Lei Zhenzi", 101401, 82}, {"Jiang Ziya", 100032, 90}, {"Ne Zha", 101674, 70}, {"Shen Gongbao", 101982, 87}, {"Jiu Weihu", 107431, 75}, {"Tian Zun", 100001, 98}, {"Tai Yi", 101009, 81}, {"Yang Jian", 101321, 63}, {"Huang Feihu", 101567, 72}, {"Zhou Wang", 108160, 55}, {"Li Jing", 102456, 84}, {"Tu Xingsun", 102245, 65}, }; int n = sizeof(students) / sizeof(Student); TreeNode* root = nullptr; for (int i = 0; i < n; i++) { root = insert(root, students[i]); } cout << "In-order traversal: " << endl; inOrder(root); cout << "Level-order traversal: " << endl; queue<TreeNode*> q; q.push(root); while (!q.empty()) { TreeNode* node = q.front(); q.pop(); if (node) { cout << node->data.name << " " << node->data.number << " " << node->data.score << endl; q.push(node->left); q.push(node->right); } } cout << "Search for Tai Yi: " << endl; TreeNode* node = search(root, "Tai Yi"); if (node) { cout << node->data.name << " " << node->data.number << " " << node->data.score << endl; } else { cout << "Not found." << endl; } cout << "Students with score <= 75: " << endl; print(root); return 0; }
时间: 2023-07-14 22:13:52 浏览: 54
以下是使用printf替换cout后的改进代码:
```c++
#include <iostream>
#include <queue>
#include <string>
using namespace std;
struct Student {
string name;
int number;
int score;
};
struct TreeNode {
Student data;
TreeNode* left;
TreeNode* right;
TreeNode(Student s) : data(s), left(nullptr), right(nullptr) {}
};
void inOrder(TreeNode* root) {
if (!root) return;
inOrder(root->left);
printf("%s %d %d\n", root->data.name.c_str(), root->data.number, root->data.score);
inOrder(root->right);
}
TreeNode* insert(TreeNode* root, Student s) {
if (!root) return new TreeNode(s);
if (s.number < root->data.number) {
root->left = insert(root->left, s);
} else {
root->right = insert(root->right, s);
}
return root;
}
TreeNode* search(TreeNode* root, string name) {
if (!root) return nullptr;
if (name == root->data.name) {
return root;
} else if (name < root->data.name) {
return search(root->left, name);
} else {
return search(root->right, name);
}
}
void print(TreeNode* root) {
if (!root) return;
if (root->data.score <= 75) {
printf("%s %d %d\n", root->data.name.c_str(), root->data.number, root->data.score);
}
print(root->left);
print(root->right);
}
int main() {
Student students[] = {
{"Lei Zhenzi", 101401, 82},
{"Jiang Ziya", 100032, 90},
{"Ne Zha", 101674, 70},
{"Shen Gongbao", 101982, 87},
{"Jiu Weihu", 107431, 75},
{"Tian Zun", 100001, 98},
{"Tai Yi", 101009, 81},
{"Yang Jian", 101321, 63},
{"Huang Feihu", 101567, 72},
{"Zhou Wang", 108160, 55},
{"Li Jing", 102456, 84},
{"Tu Xingsun", 102245, 65},
};
int n = sizeof(students) / sizeof(Student);
TreeNode* root = nullptr;
for (int i = 0; i < n; i++) {
root = insert(root, students[i]);
}
printf("In-order traversal: \n");
inOrder(root);
printf("Level-order traversal: \n");
queue<TreeNode*> q;
q.push(root);
while (!q.empty()) {
TreeNode* node = q.front();
q.pop();
if (node) {
printf("%s %d %d\n", node->data.name.c_str(), node->data.number, node->data.score);
q.push(node->left);
q.push(node->right);
}
}
printf("Search for Tai Yi: \n");
TreeNode* node = search(root, "Tai Yi");
if (node) {
printf("%s %d %d\n", node->data.name.c_str(), node->data.number, node->data.score);
} else {
printf("Not found.\n");
}
printf("Students with score <= 75: \n");
print(root);
return 0;
}
```
使用printf的好处是可以提高输出效率,特别是当需要输出大量的数据时,printf的效率要比cout高。除此之外,printf的格式化输出优势也非常明显,格式化后的输出更加美观易读。
相关推荐
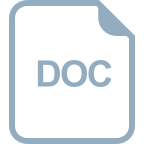
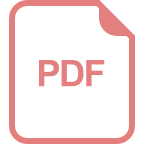
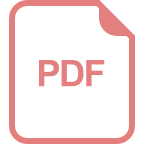















