python 生成当前日期前一年的随机dateTime()
时间: 2023-09-19 07:06:19 浏览: 66
### 回答1:
可以使用Python的datetime模块和random模块来生成当前日期前一年的随机dateTime(),代码如下:
```python
import datetime
import random
now = datetime.datetime.now() # 获取当前时间
last_year = now.year - 1 # 获取去年的年份
last_year_date = datetime.datetime(last_year, now.month, now.day) # 构造去年的日期
random_date = last_year_date + datetime.timedelta(days=random.randint(, 364)) # 随机生成去年的某一天
random_time = datetime.time(random.randint(, 23), random.randint(, 59), random.randint(, 59)) # 随机生成时间
random_datetime = datetime.datetime.combine(random_date, random_time) # 合并日期和时间
print(random_datetime) # 输出随机生成的日期时间
```
注意:以上代码仅供参考,实际应用中可能需要根据具体需求进行修改。
### 回答2:
要生成当前日期前一年的随机dateTime(),可以使用Python的random模块和datetime模块来完成。
首先,导入random和datetime模块:
import random
import datetime
然后,获取当前日期并计算出当前日期前一年的日期:
now = datetime.datetime.now()
last_year = now.year - 1
current_date = now.date()
last_year_date = datetime.date(last_year, now.month, now.day)
接下来,使用random模块生成随机的小时、分钟和秒数,并结合上一步得到的日期生成随机的时间:
random_time = datetime.time(random.randint(0, 23), random.randint(0, 59), random.randint(0, 59))
random_datetime = datetime.datetime.combine(last_year_date, random_time)
最后,输出生成的随机日期时间:
print(random_datetime)
这样就生成了当前日期前一年的随机dateTime()。注意,使用datetime模块生成的日期时间对象可以通过print语句直接输出,无需进行额外的格式化处理。
### 回答3:
要生成当前日期前一年的随机dateTime(),可以使用Python的datetime和random模块。
首先,我们需要导入datetime和random模块:
```python
import datetime
import random
```
然后,获取当前日期和时间:
```python
current_date = datetime.datetime.now()
```
接下来,计算当前日期前一年的日期。可以使用timedelta函数来实现。可以使用`-365`表示前一年:
```python
previous_year = current_date - datetime.timedelta(days=365)
```
接着,我们使用random模块的randint函数生成随机的年、月、日、时、分、秒。年范围是前一年到当前年,月范围是1到12,日范围是1到当月的最后一天,时范围是0到23,分和秒范围是0到59。
```python
random_year = random.randint(previous_year.year, current_date.year)
random_month = random.randint(1, 12)
random_day = random.randint(1, datetime.datetime(random_year, random_month+1, 1).date().day - 1)
random_hour = random.randint(0, 23)
random_minute = random.randint(0, 59)
random_second = random.randint(0, 59)
```
最后,使用datetime模块的datetime函数将随机生成的年、月、日、时、分、秒转换为dateTime对象:
```python
random_datetime = datetime.datetime(random_year, random_month, random_day, random_hour, random_minute, random_second)
```
完整的代码如下:
```python
import datetime
import random
current_date = datetime.datetime.now()
previous_year = current_date - datetime.timedelta(days=365)
random_year = random.randint(previous_year.year, current_date.year)
random_month = random.randint(1, 12)
random_day = random.randint(1, datetime.datetime(random_year, random_month+1, 1).date().day - 1)
random_hour = random.randint(0, 23)
random_minute = random.randint(0, 59)
random_second = random.randint(0, 59)
random_datetime = datetime.datetime(random_year, random_month, random_day, random_hour, random_minute, random_second)
```
通过这样的代码,我们可以生成当前日期前一年的随机dateTime()对象。
相关推荐
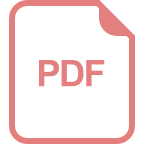
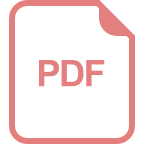














