给出一个基于pytorch的cnn-lstm程序
时间: 2023-06-02 20:02:18 浏览: 143
以下是一个基于pytorch的cnn-lstm程序的代码示例:
```
import torch
import torch.nn as nn
import torch.nn.functional as F
class CNN_LSTM(nn.Module):
def __init__(self, input_dim, hidden_dim, output_dim, num_layers=1):
super(CNN_LSTM, self).__init__()
#定义CNN层
self.conv1 = nn.Conv2d(1, 32, kernel_size=3, stride=1, padding=1)
self.conv2 = nn.Conv2d(32, 64, kernel_size=3, stride=1, padding=1)
self.pool = nn.MaxPool2d(kernel_size=2, stride=2)
#定义LSTM层
self.lstm = nn.LSTM(input_dim, hidden_dim, num_layers=num_layers, batch_first=True)
#定义全连接层
self.fc = nn.Linear(hidden_dim, output_dim)
def forward(self, x):
#CNN层
x = F.relu(self.conv1(x))
x = self.pool(x)
x = F.relu(self.conv2(x))
x = self.pool(x)
#将数据转换为LSTM的输入格式
batch_size, channels, height, width = x.size()
x = x.view(batch_size, channels, height * width)
x = x.permute(0, 2, 1)
#LSTM层
output, (h_n, c_n) = self.lstm(x)
#全连接层
output = self.fc(output[:, -1, :])
return output
```
该程序实现了一个CNN-LSTM模型,其中包含一个CNN层和一个LSTM层,最后通过全连接层输出结果。在程序中,首先定义了一个CNN层,包含两个卷积层和一个最大池化层。接着定义了一个LSTM层,包含输入维度、隐藏层维度、输出维度和层数等参数。最后定义了一个全连接层,将LSTM层的输出映射到最终的结果。在forward方法中,先通过CNN层对输入数据进行卷积和池化操作,将数据转换为LSTM层的输入格式,然后通过LSTM层和全连接层得到最终的输出结果。
阅读全文
相关推荐
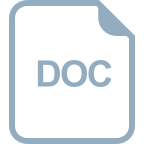
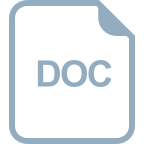
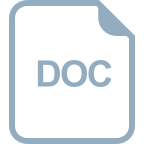









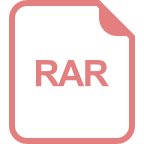
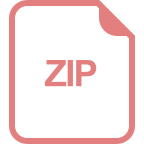
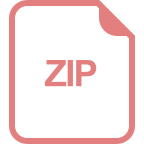



