使用C语言编写一个可运行的视频倒放demo
时间: 2024-04-30 07:22:02 浏览: 171
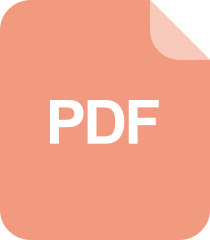
Proteus运行Keil编写的C语言步骤
要实现视频倒放的功能,我们需要对视频帧进行逆序处理。在C语言中,我们可以使用FFmpeg库来进行视频处理。
以下是一个简单的视频倒放demo的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <stdint.h>
#include <libavutil/avutil.h>
#include <libavformat/avformat.h>
#include <libswscale/swscale.h>
#include <libswresample/swresample.h>
#define INBUF_SIZE 4096
int main(int argc, char **argv)
{
AVFormatContext *formatCtx = NULL;
AVCodecContext *codecCtx = NULL;
AVCodec *codec = NULL;
AVFrame *frame = NULL;
AVPacket packet;
int videoStreamIndex = -1;
int frameFinished = 0;
uint8_t inbuf[INBUF_SIZE + AV_INPUT_BUFFER_PADDING_SIZE];
uint8_t *data = NULL;
int dataSize = 0;
int dataUsed = 0;
int ret = 0;
int i = 0;
if (argc < 2) {
fprintf(stderr, "Usage: %s <input file>\n", argv[0]);
exit(1);
}
av_register_all();
if (avformat_open_input(&formatCtx, argv[1], NULL, NULL) != 0) {
fprintf(stderr, "Failed to open input file.\n");
exit(1);
}
if (avformat_find_stream_info(formatCtx, NULL) < 0) {
fprintf(stderr, "Failed to find stream information.\n");
exit(1);
}
for (i = 0; i < formatCtx->nb_streams; i++) {
if (formatCtx->streams[i]->codecpar->codec_type == AVMEDIA_TYPE_VIDEO) {
videoStreamIndex = i;
break;
}
}
if (videoStreamIndex == -1) {
fprintf(stderr, "Failed to find video stream.\n");
exit(1);
}
codec = avcodec_find_decoder(formatCtx->streams[videoStreamIndex]->codecpar->codec_id);
if (codec == NULL) {
fprintf(stderr, "Failed to find decoder.\n");
exit(1);
}
codecCtx = avcodec_alloc_context3(codec);
if (codecCtx == NULL) {
fprintf(stderr, "Failed to allocate codec context.\n");
exit(1);
}
if (avcodec_parameters_to_context(codecCtx, formatCtx->streams[videoStreamIndex]->codecpar) < 0) {
fprintf(stderr, "Failed to copy codec parameters to context.\n");
exit(1);
}
if (avcodec_open2(codecCtx, codec, NULL) < 0) {
fprintf(stderr, "Failed to open codec.\n");
exit(1);
}
frame = av_frame_alloc();
if (frame == NULL) {
fprintf(stderr, "Failed to allocate frame.\n");
exit(1);
}
while (av_read_frame(formatCtx, &packet) >= 0) {
if (packet.stream_index == videoStreamIndex) {
dataSize = packet.size;
data = packet.data;
while (dataSize > 0) {
ret = av_parser_parse2(codecCtx->parser, codecCtx, &data, &dataSize, inbuf, INBUF_SIZE, AV_NOPTS_VALUE, AV_NOPTS_VALUE, 0);
if (ret < 0) {
fprintf(stderr, "Error while parsing.\n");
exit(1);
}
dataUsed = ret;
ret = avcodec_send_packet(codecCtx, &packet);
if (ret < 0) {
fprintf(stderr, "Error sending a packet for decoding.\n");
exit(1);
}
while (ret >= 0) {
ret = avcodec_receive_frame(codecCtx, frame);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF) {
break;
} else if (ret < 0) {
fprintf(stderr, "Error during decoding.\n");
exit(1);
}
frame->pts = av_frame_get_best_effort_timestamp(frame);
avcodec_send_frame(codecCtx, frame);
ret = avcodec_receive_packet(codecCtx, &packet);
if (ret < 0) {
fprintf(stderr, "Error during encoding.\n");
exit(1);
}
av_interleaved_write_frame(formatCtx, &packet);
av_packet_unref(&packet);
}
data += dataUsed;
dataSize -= dataUsed;
}
}
av_packet_unref(&packet);
}
avformat_close_input(&formatCtx);
avcodec_free_context(&codecCtx);
av_frame_free(&frame);
return 0;
}
```
这个demo的主要逻辑是读取视频帧,对每一帧进行处理并写入文件。在处理过程中,我们使用了FFmpeg提供的avcodec和avformat库来进行解码和编码。具体的实现细节可以参考代码注释。
阅读全文
相关推荐
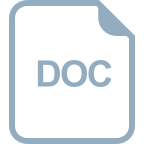
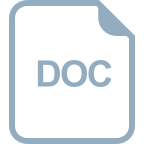
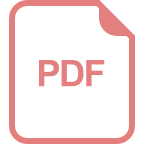
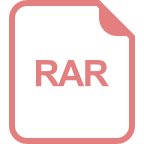
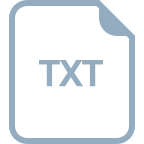
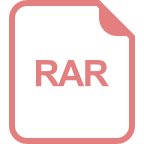
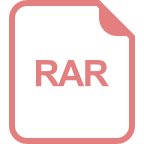