// 监听表单提交事件 $('#form').submit(function(event) { // 阻止表单默认提交行为 event.preventDefault(); // 创建新的请求链接 var newUrl = "UserController?action=drawCode&" + new Date(); // 发送 AJAX 请求 $.ajax({ url: newUrl, method: 'GET', success: function(data) { // 成功响应后更新验证码图片 $('#img').attr('src', newUrl); }, error: function(error) { console.log('Error:', error); } }); });这串代码该写在那个里面
时间: 2024-04-26 12:20:32 浏览: 194
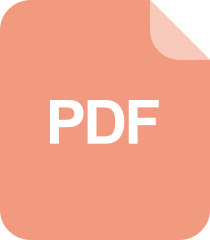
jquery实现预览提交的表单代码分享
这段代码应该写在一个 JavaScript 文件中,并且需要在 HTML 页面中引入该 JavaScript 文件。可以将该代码写在 `<script>` 标签中,或者在外部定义一个 `.js` 文件并使用 `<script src="yourfile.js"></script>` 引入。
同时,要确保在 HTML 页面中存在一个 `id` 为 `form` 的表单元素和一个 `id` 为 `img` 的图片元素,以便在表单提交时更新验证码图片。
阅读全文
相关推荐
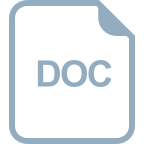
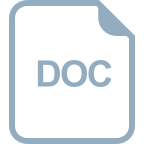
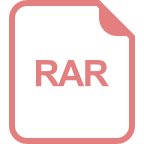













请把一下代码每一行的用途注释上:<!DOCTYPE html> <html> <head> <meta charset="utf-8" /> <title>登录表单</title> <style> body { background-image: url("background.jpg"); background-size: cover; font-family: "宋体"; font-size: 30px; } form { margin: auto; width: 50%; background-color: rgba(255,255,255,0.8); padding: 20px; border-radius: 10px; } input[type=text], input[type=password] { width: 100%; padding: 12px 20px; margin: 8px 0; display: inline-block; border: 1px solid #ccc; border-radius: 4px; box-sizing: border-box; } input[type=submit], input[type=reset] { background-color: #4CAF50; color: white; padding: 14px 20px; margin: 8px 0; border: none; border-radius: 4px; cursor: pointer; } input[type=submit]:hover, input[type=reset]:hover { background-color: #45a049; } .error { color: red; } </style> </head> <body> <form> 登录 <label for="username">用户名</label> <input type="text" id="username" name="username" >
<label for="password">密码</label> <input type="password" id="password" name="password" >
<label for="confirm_password">确认密码</label> <input type="password" id="confirm_password" name="confirm_password" >
<input type="submit" value="登录"> <input type="reset" value="重置">
忘记密码? </form> <script> document.querySelector("form").addEventListener("submit", function(event) { var password = document.querySelector("#password").value; var confirm_password = document.querySelector("#confirm_password").value; if (password != confirm_password) { document.querySelector("#password_error").innerHTML = "密码不一致"; event.preventDefault(); } }); </script> </body> </html>

