机械臂逆运动学如何写C语言
时间: 2023-09-27 15:08:23 浏览: 49
机械臂的逆运动学一般使用解析法或数值法来求解,其中解析法比较复杂,数值法相对简单,但计算速度较慢。以下是一个简单的数值法的C语言代码示例:
```c
#include <stdio.h>
#include <math.h>
#define PI 3.1415926
// 机械臂各关节长度
const double L1 = 1.0;
const double L2 = 1.0;
const double L3 = 1.0;
// 目标位置坐标
const double x = 1.5;
const double y = 0.5;
const double z = 0.5;
// 初始角度
double theta1 = 0.0;
double theta2 = 0.0;
double theta3 = 0.0;
// 计算两点之间的距离
double distance(double x1, double y1, double z1, double x2, double y2, double z2) {
return sqrt(pow(x1 - x2, 2) + pow(y1 - y2, 2) + pow(z1 - z2, 2));
}
int main() {
double d, err;
double dx, dy, dz;
int i;
for (i = 0; i < 100; i++) { // 最多迭代100次
// 计算当前位置
double x0 = L1 * cos(theta1) * cos(theta2) + L2 * cos(theta1) * cos(theta2 + theta3) + L3 * cos(theta1) * cos(theta2 + theta3);
double y0 = L1 * sin(theta1) * cos(theta2) + L2 * sin(theta1) * cos(theta2 + theta3) + L3 * sin(theta1) * cos(theta2 + theta3);
double z0 = L1 * sin(theta2) + L2 * sin(theta2 + theta3) + L3 * sin(theta2 + theta3);
// 计算目标位置与当前位置之间的距离
d = distance(x0, y0, z0, x, y, z);
if (d < 0.001) { // 如果距离小于误差范围,认为已经到达目标位置
break;
}
// 计算目标位置与当前位置之间的方向向量
dx = (x - x0) / d;
dy = (y - y0) / d;
dz = (z - z0) / d;
// 计算旋转角度
theta1 += 0.01 * dz;
theta2 += 0.01 * (dx * sin(theta1) - dy * cos(theta1));
theta3 += 0.01 * (dx * cos(theta1) * sin(theta2) + dy * sin(theta1) * sin(theta2) - dz * cos(theta2));
// 将角度限制在合理范围内
if (theta1 > 2 * PI) {
theta1 -= 2 * PI;
} else if (theta1 < 0) {
theta1 += 2 * PI;
}
if (theta2 > 2 * PI) {
theta2 -= 2 * PI;
} else if (theta2 < 0) {
theta2 += 2 * PI;
}
if (theta3 > 2 * PI) {
theta3 -= 2 * PI;
} else if (theta3 < 0) {
theta3 += 2 * PI;
}
}
// 输出结果
printf("theta1 = %lf\n", theta1);
printf("theta2 = %lf\n", theta2);
printf("theta3 = %lf\n", theta3);
return 0;
}
```
注:以上代码仅为示例,实际应用中还需要考虑各关节的初始角度、角度限制范围等问题。
相关推荐
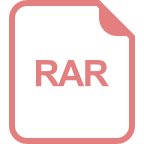










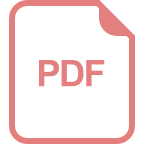
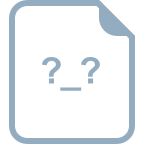