提示用户输入一个文件名,显示该文件名中每个字母出现的次数,字母区分大小写
时间: 2024-10-13 09:07:44 浏览: 14
为了实现这个功能,你需要通过Scanner获取用户输入的文件名,然后逐字符读取文件内容统计字母出现次数。以下是使用Java的一个简单示例:
首先,导入需要的包:
```java
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
```
接着,编写主函数:
```java
public class CharacterFrequencyCounter {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("请输入文件名:");
String fileName = scanner.nextLine();
try {
countCharactersInFile(fileName);
} catch (FileNotFoundException e) {
System.out.println("文件未找到: " + e.getMessage());
}
scanner.close();
}
private static void countCharactersInFile(String fileName) throws FileNotFoundException {
File file = new File(fileName);
int[] frequency = new int[26]; // 26 for 'a' to 'z' and 'A' to 'Z'
int totalLines = 0;
try (BufferedReader reader = new BufferedReader(new FileReader(file))) {
String line;
while ((line = reader.readLine()) != null) {
totalLines++;
for (char c : line.toCharArray()) {
if (Character.isLetter(c)) { // 只统计字母
char lowerCaseChar = Character.toLowerCase(c); // 转换为小写便于统一计数
frequency[lowerCaseChar - 'a']++; // 更新相应字母的频率
}
}
}
}
printCharacterFrequencies(frequency, totalLines);
}
private static void printCharacterFrequencies(int[] frequencies, int totalLines) {
System.out.println("文件 " + fileName + " 的统计结果:");
for (int i = 0; i < 26; i++) {
char letter = (char) ('a' + i);
if (frequencies[i] > 0) {
System.out.printf("%s 出现了 %d 次, 占总字符数的 %.2f%%\n", letter, frequencies[i], (double) frequencies[i] / totalLines * 100);
} else {
System.out.print(letter + " 未出现");
}
}
}
}
```
当你运行这个程序时,它会提示用户输入文件名,然后计算每个字母在文件中出现的次数,并按照百分比显示。
阅读全文
相关推荐
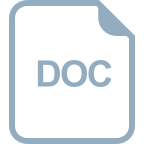
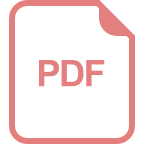
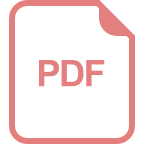

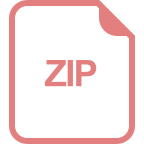
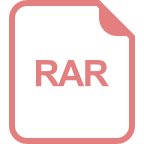
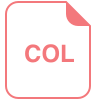
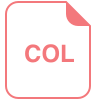











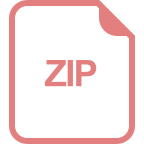