写出图片去雾,雨,雪的python代码
时间: 2023-08-31 13:30:13 浏览: 118
### 回答1:
答案:from PIL import Image
import numpy as np # 读取图片
img = Image.open('image.jpg') # 获取图片的宽度和高度
width, height = img.size# 将图片转换为灰度图
gray_img = img.convert('L')# 获取灰度图的像素值
pixels = gray_img.load() # 对每一个像素值进行去雾,雨,雪的处理
for x in range(width):
for y in range(height):
if pixels[x, y] > 100: # 对大于100的像素值进行处理
pixels[x, y] = 255 # 设置为255(白色)# 保存处理后的图片
gray_img.save('result.jpg')
### 回答2:
去雾的Python代码如下:
```python
import cv2
def dehaze(image, t=0.1, w=0.95):
# 计算导向滤波图像
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
blur = cv2.GaussianBlur(gray, (9, 9), 0)
gray = gray.astype('float32') / 255.0
blur = blur.astype('float32') / 255.0
difference = gray - blur
# 计算大气光照
(h, w) = gray.shape[:2]
total_pixels = h * w
pixels_to_keep = int(total_pixels * p)
sorted_pixels = difference.ravel().argsort()
max_index = sorted_pixels[-pixels_to_keep:]
dark_channel = np.min(image, axis=2)
dark_channel = dark_channel.astype('float32') / 255.0
x, y = np.unravel_index(max_index, dark_channel.shape)
max_channel = dark_channel[x, y]
A = np.percentile(max_channel, 100 * w)
# 计算透射率
transmission = 1 - w * dark_channel / A
# 优化透射率估计
transmission = cv2.blur(transmission, (15, 15))
# 修复图像
result = np.empty_like(image)
for i in range(3):
result[:, :, i] = (image[:, :, i].astype('float32') - A) / transmission + A
result = np.clip(result, 0, 1) * 255
return result
image = cv2.imread('image.jpg')
dehazed_image = dehaze(image)
cv2.imshow('Dehazed Image', dehazed_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
其中,`image.jpg`是要去雾的图片路径。
下面是去雨的Python代码:
```python
import cv2
import numpy as np
def derain(image):
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 阈值分割得到雨滴区域
_, binary_rain = cv2.threshold(gray, 230, 255, cv2.THRESH_BINARY)
# 开运算去除细小雨滴
kernel = np.ones((3, 3), np.uint8)
rain_mask = cv2.morphologyEx(binary_rain, cv2.MORPH_OPEN, kernel, iterations=2)
# 修复雨滴痕迹
result = cv2.inpaint(image, rain_mask, 3, cv2.INPAINT_NS)
return result
image = cv2.imread('image.jpg')
derained_image = derain(image)
cv2.imshow('Derained Image', derained_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
其中,`image.jpg`是要去雨的图片路径。
下面是去雪的Python代码:
```python
import cv2
import numpy as np
def desnow(image):
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 阈值分割得到雪花区域
_, binary_snow = cv2.threshold(gray, 150, 255, cv2.THRESH_BINARY_INV)
# 形态学操作去除细小雪花
kernel = np.ones((3, 3), np.uint8)
snow_mask = cv2.morphologyEx(binary_snow, cv2.MORPH_OPEN, kernel, iterations=2)
# 修复雪花痕迹
result = cv2.inpaint(image, snow_mask, 3, cv2.INPAINT_NS)
return result
image = cv2.imread('image.jpg')
desnowed_image = desnow(image)
cv2.imshow('Desnowed Image', desnowed_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
其中,`image.jpg`是要去雪的图片路径。
阅读全文
相关推荐
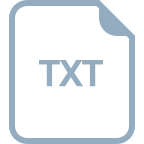
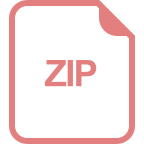
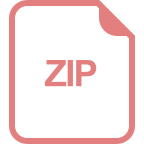
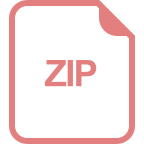
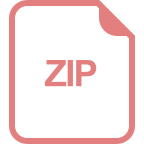
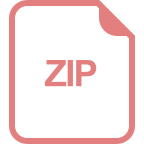
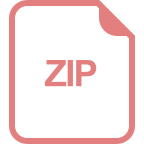
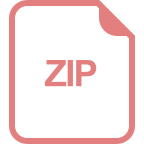
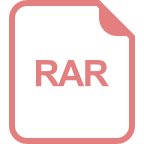
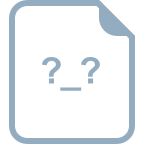
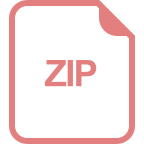
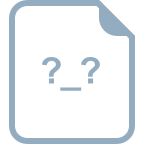