springboot中自定义异步线程池
时间: 2023-08-31 18:24:51 浏览: 71
### 回答1:
在Spring Boot中,我们可以通过自定义异步线程池来优化应用程序的性能。下面是一个简单的示例,演示如何在Spring Boot应用程序中自定义异步线程池:
1. 首先,我们需要在Spring Boot应用程序的配置文件中定义异步线程池的属性:
```
spring.task.execution.pool.core-size=10
spring.task.execution.pool.max-size=20
spring.task.execution.pool.queue-capacity=1000
```
上述配置定义了异步线程池的核心大小、最大大小和队列容量。
2. 然后,我们需要创建一个自定义的异步线程池配置类,如下所示:
```
@Configuration
@EnableAsync
public class AsyncConfig implements AsyncConfigurer {
@Override
public Executor getAsyncExecutor() {
ThreadPoolTaskExecutor executor = new ThreadPoolTaskExecutor();
executor.setCorePoolSize(10);
executor.setMaxPoolSize(20);
executor.setQueueCapacity(1000);
executor.setThreadNamePrefix("MyCustomExecutor-");
executor.initialize();
return executor;
}
}
```
上述配置类实现了AsyncConfigurer接口,并覆盖了getAsyncExecutor()方法,该方法返回一个自定义的ThreadPoolTaskExecutor对象,其中设置了异步线程池的核心大小、最大大小、队列容量和线程名称前缀。
3. 最后,在需要异步执行的方法上添加@Async注解即可:
```
@Service
public class MyService {
@Async
public void doSomethingAsync() {
// 异步执行的代码
}
}
```
上述示例中,我们在MyService类的doSomethingAsync()方法上添加了@Async注解,表示该方法需要异步执行。
这样,我们就成功地自定义了Spring Boot应用程序中的异步线程池。
### 回答2:
在Spring Boot中,可以通过配置来自定义异步线程池。我们可以利用`@EnableAsync`注解开启异步支持,并使用`@Async`注解来标识需要异步执行的方法。
首先,在Spring Boot的配置文件(如application.properties或application.yml)中配置线程池的相关属性,例如线程池核心线程数、最大线程数、队列容量等。示例配置如下:
```
spring.task.execution.pool.core-size=10
spring.task.execution.pool.max-size=20
spring.task.execution.pool.queue-capacity=100
```
接下来,在启动类上添加`@EnableAsync`注解,以开启异步支持。例如:
```java
@SpringBootApplication
@EnableAsync
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
```
然后,我们可以在需要异步执行的方法上添加`@Async`注解,以告知Spring该方法需要异步执行。例如:
```java
@Service
public class MyService {
@Async
public void asyncMethod() {
// 异步执行的方法体
}
}
```
在上述示例中,`asyncMethod()`方法会被Spring框架异步执行,Spring会自动从线程池中获取一个线程来执行该方法。
通过以上步骤,我们就可以在Spring Boot中自定义异步线程池。我们可以根据具体的业务需求来调整线程池的配置,以达到最佳的性能和资源利用效果。
相关推荐
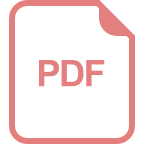
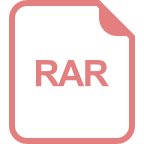














