list_first_entry_or_null
时间: 2023-08-06 08:02:36 浏览: 111
`list_first_entry_or_null` 是一个宏定义在 Linux 内核中,用于获取链表的第一个元素的指针,如果链表为空则返回 NULL。
它的定义如下:
```c
#define list_first_entry_or_null(ptr, type, member) \
(!list_empty(ptr) ? list_first_entry(ptr, type, member) : NULL)
```
与 `list_first_entry` 宏类似,`ptr` 是链表头指针,`type` 是链表节点的数据类型,`member` 是链表节点在数据结构中的成员名。
使用 `list_first_entry_or_null` 宏可以安全地获取链表的第一个节点的指针,即使链表为空,也会返回 NULL 而不会导致错误。这在需要处理可能为空的链表时很有用。
相关问题
这段代码是什么意思 cmeq_link_t* get_link_from_pool() { cmeq_link_t *plink = NULL; pthread_mutex_lock(&link_pool.lock_idle); while (list_empty(&link_pool.idle_head)) { pthread_mutex_unlock(&link_pool.lock_idle); sem_wait(&link_pool.wait_idle_link); pthread_mutex_lock(&link_pool.lock_idle); } plink = list_first_entry(&link_pool.idle_head, cmeq_link_t, user); list_del_init(&(plink->user)); pthread_mutex_unlock(&link_pool.lock_idle); // plink->testFlg = 1; //printf("get cmeq link %d\n", ++link_cnt); return plink; }
这段代码是一个函数,函数名为get_link_from_pool,返回值为cmeq_link_t类型的指针。这个函数的作用是从连接池中获取一个空闲的连接,并返回该连接的指针。
在函数中,首先定义了一个空指针plink,然后通过pthread_mutex_lock函数锁定了连接池的空闲连接列表。接下来使用while循环,在连接池的空闲连接列表为空的情况下,使用sem_wait函数等待连接池中有空闲连接可用。当获取到一个空闲连接时,使用list_first_entry函数获取该连接,并从空闲连接列表中删除该连接。最后通过pthread_mutex_unlock函数解锁连接池的空闲连接列表,并将获取到的连接指针返回。
注释中的plink->testFlg = 1和printf语句是注释掉的代码,不会被执行。
Failed to execute script 'entry_point' due to unhandled exception!
引用\[1\]:当遇到"Failed to execute script 'entry_point' due to unhandled exception"错误时,可能有几个原因导致。首先,检查是否有缺少必要的模块或库,可能需要安装或更新相关的依赖项。其次,确保代码中没有导入错误,比如引用了不存在的模块或函数。还要检查是否有文件路径错误或文件损坏的问题。最后,还要确保系统环境变量设置正确,特别是PYTHONPATH变量。\[1\]\[2\]
引用\[3\]:另外,如果遇到"Failed to execute script 'entry_point' due to unhandled exception"错误,也可能是因为系统环境配置问题。例如,在Ubuntu 20.04 server上安装了Python3.9,但在修改默认Python版本时,不小心删除了/usr/bin/python3软连接,导致某些文件无法找到对应的Python版本。解决方法是将系统原文件/usr/lib/python3/dist-packages/lsb_release.py复制到/usr/bin目录下,可以使用命令sudo cp /usr/lib/python3/dist-packages/lsb_release.py /usr/bin来完成。\[3\]
综上所述,当遇到"Failed to execute script 'entry_point' due to unhandled exception"错误时,可以通过检查缺少的模块、导入错误、文件路径错误、文件损坏以及系统环境配置等方面来解决问题。
#### 引用[.reference_title]
- *1* *2* [Failed to execute script ‘first‘ due to unhandled exception:cannot import name问题](https://blog.csdn.net/wm9028/article/details/124527206)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^control_2,239^v3^insert_chatgpt"}} ] [.reference_item]
- *3* [Failed to execute script entry_point与Command ‘lsb_release -a‘ returned non-zero错误-安装miniconda...](https://blog.csdn.net/qq_55059421/article/details/126333811)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^control_2,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
相关推荐
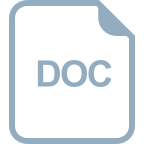
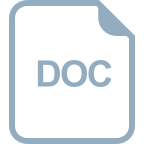
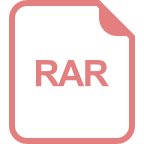












