MATLAB软件(或者Python 、C++软件)编程实现下列算法: 1.中点画椭圆;并用编好的程序实现画椭圆(也就是举例子)。
时间: 2024-11-22 17:35:21 浏览: 11
MATLAB是一个强大的数学计算和图形处理工具,常用于科学计算和工程应用。要在MATLAB中实现绘制椭圆,你可以使用`ellipse`函数,这是一个内置的绘图函数,可以直接生成椭圆。
Python方面,虽然Python的标准库并没有直接提供类似的功能,但我们可以利用matplotlib等第三方库来绘制。以下是一个简单的例子:
```python
import matplotlib.pyplot as plt
from matplotlib.patches import Ellipse
# 定义椭圆中心点和大小
center = (0, 0) # 椭圆中心坐标
width = 5 # 长轴半径
height = 3 # 短轴半径
# 创建椭圆对象
ellipse = Ellipse(center, width, height, fill=False)
# 绘制椭圆
fig, ax = plt.subplots()
ax.add_patch(ellipse)
ax.set_xlim(-10, 10)
ax.set_ylim(-10, 10)
plt.show()
```
在C++中,可以借助开源的图形库如SFML或者OpenGL来绘制,但这需要编写更多的底层代码。例如使用SFML:
```cpp
#include <SFML/Graphics.hpp>
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "Eclipse");
sf::CircleShape ellipse(40.f); // 使用圆形近似椭圆,因为C++标准库无现成椭圆形状
ellipse.setPosition(window.getSize().x / 2, window.getSize().y / 2);
ellipse.setFillColor(sf::Color::Black);
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
window.draw(ellipse);
window.display();
}
return 0;
}
```
阅读全文
相关推荐
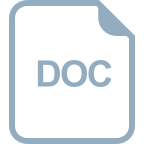
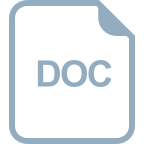
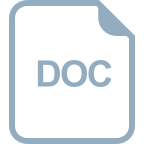
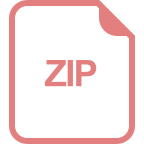
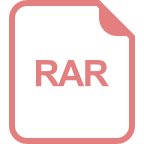
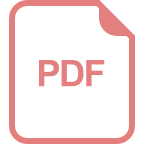
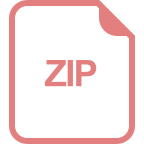
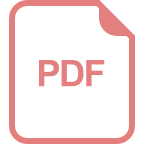
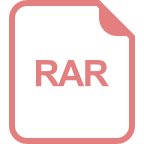
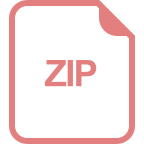
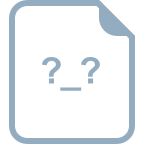
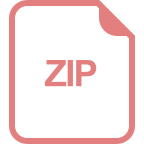
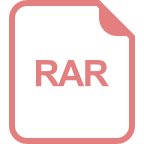
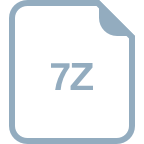
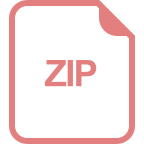
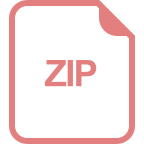
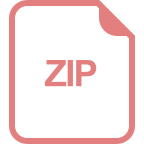
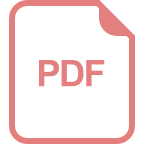