与国庆动画有关的C语言代码,用Dev-c++软件运行
时间: 2024-09-29 09:07:11 浏览: 21
在C语言中编写与国庆相关的动画程序并用Dev-C++软件运行可能会涉及到图形界面编程,通常我们会选择Windows API函数或者使用一些轻量级的游戏引擎库如SFML。由于C标准库并不直接支持动画绘制,所以这里我会给你提供一个简单的例子,使用ASCII艺术来模拟国庆节烟花效果。
```c
#include <stdio.h>
#include <windows.h> // 包含Windows API函数
// 动画函数,每秒更新一次屏幕
void drawFireworks(int count) {
for (int i = 0; i < count; ++i) {
printf(" * ");
Sleep(100); // 暂停100毫秒模拟烟花绽放时间
system("cls"); // 清屏,显示新的烟花位置
}
}
int main() {
const int fireworks_count = 50;
drawFireworks(fireworks_count);
return 0;
}
```
在这个代码中,我们定义了一个`drawFireworks`函数,通过循环打印出星星(*)来模拟烟花,每次暂停一会儿再清空屏幕,看起来就像是烟花在变化。然后在`main`函数里调用这个函数,设置一定的烟花数量。
**注意**: `Sleep(100)`会使程序暂停一段时间,对于动画效果可能不够流畅。在实际项目中,可以考虑使用更专业的图形库或者定时器来进行动画控制。
相关问题
c++国庆节课程代码
在C++中编写国庆节相关的课程代码,通常可以创建一个小程序来模拟庆祝活动或者展示节日祝福。下面是一个简单的例子,它是一个控制台程序,当用户运行时会显示国庆节的问候:
```cpp
#include <iostream>
#include <ctime> // 包含时间库以便获取当前日期
int main() {
// 获取当前时间并计算是否是中国的国庆日(10月1日)
std::time_t now = std::time(0);
std::tm* current_time = std::localtime(&now);
if (current_time->tm_mday == 1 && current_time->tm_mon + 1 == 10) { // 10月1日
std::cout << "国庆快乐!今天是中华人民共和国的生日!" << std::endl;
} else {
std::cout << "当前不是国庆节,不过还是祝你愉快!" << std::endl;
}
return 0;
}
```
c语言 国庆节快乐打印
C语言是一种广泛应用于计算机编程的高级编程语言。它具有简洁高效的特点,被广泛用于各种领域。在国庆节这个特殊的日子里,我们可以利用C语言来编写一个简单的程序,实现打印“国庆节快乐”的功能。
首先,我们使用C语言的输入输出函数printf来打印字符串。在C语言中,我们可以用双引号括起来的字符串字面量来表示一个字符串。因此,我们可以使用printf函数打印出字符串“国庆节快乐”。
下面是一个示例代码:
#include <stdio.h>
int main() {
printf("国庆节快乐!\n");
return 0;
}
在这段代码中,我们通过#include指令导入了stdio.h头文件,其中定义了printf函数。主函数main中包含了printf函数,它打印了字符串“国庆节快乐!”并通过换行符\n来换行。
当我们编译并运行这段代码时,屏幕上将会打印出“国庆节快乐!”。这是一个简单的C语言程序,通过printf函数来实现打印“国庆节快乐”的功能。
希望大家在国庆节期间玩得开心,快乐幸福!
相关推荐
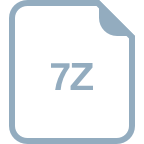
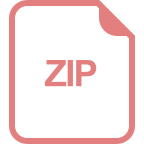
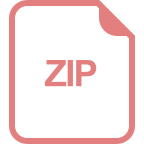
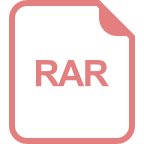
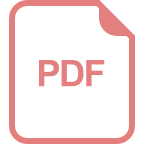
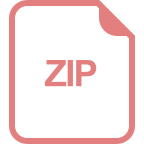
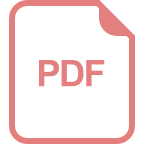
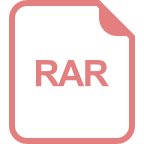
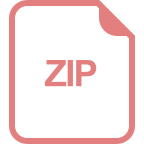
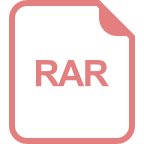
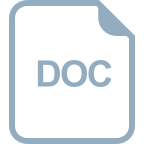
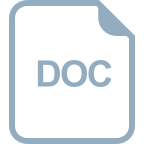