爬取网易云音乐歌单的代码
时间: 2024-08-13 07:07:50 浏览: 59
可以使用Python的Selenium库和BeautifulSoup库来爬取网易云音乐歌单。以下是一个示例代码:
```python
from selenium import webdriver
from bs4 import BeautifulSoup
# 设置Chrome浏览器的驱动路径
driver_path = 'chromedriver.exe'
driver = webdriver.Chrome(driver_path)
# 打开网易云音乐歌单页面
url = 'https://music.163.com/playlist?id=90771773'
driver.get(url)
# 切换到iframe框架
driver.switch_to.frame('contentFrame')
# 获取歌单页面的HTML内容
html = driver.page_source
# 解析HTML内容,获取歌曲名称列表
soup = BeautifulSoup(html, 'lxml')
song_list = soup.find_all('span', {'class': 'txt'})
# 输出歌曲名称列表
for song in song_list:
print(song.text)
# 关闭浏览器
driver.quit()
```
该代码使用Chrome浏览器驱动打开网易云音乐歌单页面,然后切换到iframe框架,获取歌单页面的HTML内容,并使用BeautifulSoup库解析HTML内容,获取歌曲名称列表。最后输出歌曲名称列表。
相关问题
用selenium爬取网易云音乐歌单并且保存为csv文件
首先,你需要安装 Selenium 库,并且需要一个可以运行的浏览器驱动。下面是一个示例代码,它会爬取网易云音乐歌单的歌曲名称和歌手名称,并将这些信息保存到 CSV 文件中:
```python
# 导入必要的库
from selenium import webdriver
from selenium.webdriver.common.by import By
import csv
# 定义保存到 CSV 文件中的信息的列表
songs = []
# 使用 webdriver 启动浏览器
driver = webdriver.Firefox()
# 使用浏览器访问网易云音乐歌单的 URL
driver.get("https://music.163.com/#/playlist?id=你的歌单 ID")
# 等待页面加载完成
driver.implicitly_wait(10)
# 获取歌曲列表的 div 元素
song_list_div = driver.find_element(By.ID, "song-list-pre-cache")
# 获取所有的 li 元素
li_elements = song_list_div.find_elements(By.TAG_NAME, "li")
# 遍历所有的 li 元素
for li in li_elements:
# 获取歌曲名称和歌手名称
song_name = li.find_element(By.CLASS_NAME, "f-thide").text
artist_name = li.find_element(By.CLASS_NAME, "s-fc3").text
# 将歌曲信息添加到列表中
songs.append((song_name, artist_name))
# 关闭浏览器
driver.close()
# 将歌曲信息写入 CSV 文件中
with open("songs.csv", "w", newline="") as csvfile:
writer = csv.writer(csvfile)
writer.writerows(songs)
```
在这段代码中,我们使用了 Selenium 的 webdriver 模块启动了 Firefox 浏
selenium爬取网易云歌单创作者
以下是使用Python Selenium爬取网易云音乐歌单创作者的示例代码:
```python
from selenium import webdriver
# 创建浏览器对象
browser = webdriver.Chrome()
# 打开网易云音乐歌单页面
browser.get('https://music.163.com/#/playlist?id=歌单ID')
# 切换到iframe
browser.switch_to.frame('contentFrame')
# 获取歌单创作者
creator = browser.find_element_by_css_selector('.user.f-ff2.s-fc3').text
# 输出歌单创作者
print('歌单创作者:', creator)
# 关闭浏览器
browser.quit()
```
其中,需要将代码中的“歌单ID”替换为实际的歌单ID。可以通过在网易云音乐中打开歌单页面,查看URL中的“id=”后面的数字即为歌单ID。
相关推荐
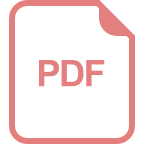
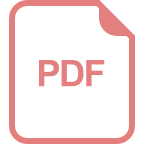
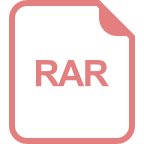











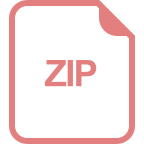
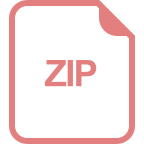