Java 发送SOAP请求调用WebService,解析SOAP报文
时间: 2024-10-15 09:15:22 浏览: 64
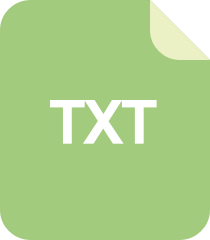
接口输入报文
在Java中,发送SOAP请求并处理Web Service通常涉及以下几个步骤:
1. **添加依赖**:首先需要引入JAX-RPC(Java API for XML Remote Proceding Calls)或者更现代的JAXB(Java Architecture for XML Binding)以及SAAJ(Simple API for XML Serialization and deserialization)库,它们都是为了处理SOAP相关的操作。
2. **创建SOAP消息**:使用JAXB可以将Java对象转换成XML SOAP消息,也可以直接使用`javax.xml.soap.SOAPMessageFactory`创建SOAP消息。
```java
SOAPConnectionFactory soapConnectionFactory = SOAPConnectionFactory.newInstance();
SOAPMessage message = soapConnectionFactory.createMessage();
SOAPPart soapPart = message.getSOAPPart();
MimeHeaders headers = soapPart.getMimeHeaders();
headers.addHeader(new HeaderImpl("To", "http://webservice.example.com/service"));
```
3. **设置SOAPAction和SOAP Body**:
```java
SOAPEnvelope envelope = soapPart.getEnvelope();
SOAPBody body = envelope.getBody();
SOAPElement bodyElem = body.addChildElement("serviceCall", "ns", "http://example.com");
ObjectFactory objectFactory = new ObjectFactory();
YourRequest requestObj = ...; // 创建你要发送的对象
JAXBElement<YourRequest> reqElement = objectFactory.createYourRequest(requestObj);
bodyElem.addChildElement(reqElement);
```
4. **发送请求**:使用HTTP客户端库如Apache HttpClient或者Java内置的URLConnection发送POST请求到指定的URL。
5. **接收响应**:服务器返回SOAP响应后,同样使用JAX-RPC或JAXB将其解析成Java对象。
6. **解析SOAP报文**:
```java
String responseString = ...; // 获取服务器响应字符串
SOAPMessage receivedMessage = MessageFactory.newInstance().createMessage();
receivedMessage.importFrom(new StreamSource(new ByteArrayInputStream(responseString.getBytes())));
SOAPEnvelope envelope = receivedMessage.getSOAPPart().getEnvelope();
// 解析body中的数据
SOAPBody body = envelope.getBody();
SOAPElement responseElement = body.getChildElements().next();
YourResponse responseObj = ...; // 根据responseElement解析结果
```
阅读全文
相关推荐
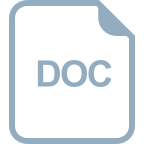
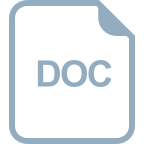
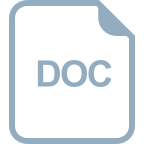


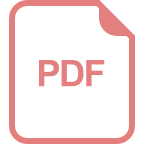
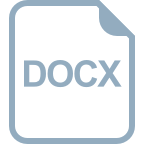
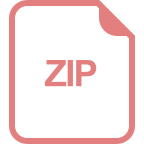
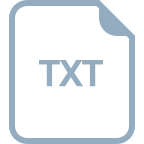
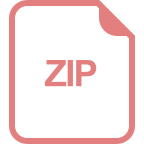
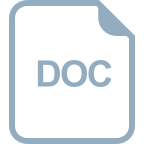






