Qt c++解析xml文件
时间: 2024-10-10 11:01:02 浏览: 48
在Qt C++中,可以使用内置的Qt XML模块(如`QDomDocument`和`QDomElement`)来解析XML文件。以下是基本步骤:
1. **包括头文件**:
```cpp
#include <QDomDocument>
#include <QFile>
```
2. **创建并打开文件**:
```cpp
QFile xmlFile("path_to_your_xml_file.xml");
if (!xmlFile.open(QIODevice::ReadOnly)) {
// 处理文件打开错误
}
```
3. **创建DOM解析器**:
```cpp
QDomDocument doc;
if (!doc.setContent(xmlFile.readAll())) {
// 解析失败,查看错误信息
}
```
4. **获取根节点**:
```cpp
QDomNode root = doc.documentElement();
```
5. **遍历和访问节点**:
```cpp
QDomElement child = root.firstChildElement(); // 获取第一个子元素
while (!child.isNull()) {
QDomAttr attr = child.attribute("attribute_name"); // 访问属性
qDebug() << child.tagName() << " - " << attr.value();
child = child.nextSiblingElement(); // 移动到下一个兄弟元素
}
```
6. **完成后关闭文件**:
```cpp
xmlFile.close();
```
记得处理可能出现的异常情况,如文件读取、解析失败等。
阅读全文
相关推荐
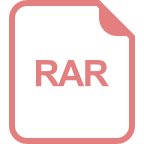
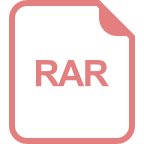
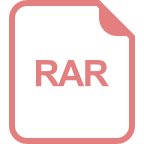
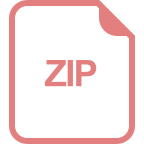
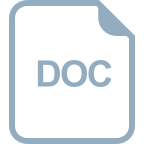
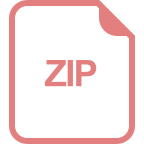
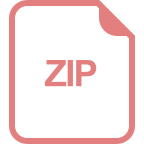
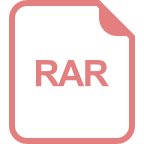
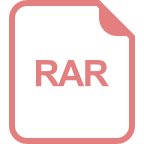
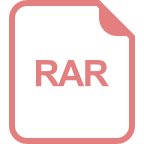
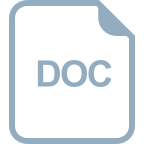
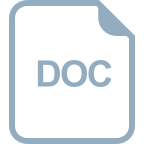
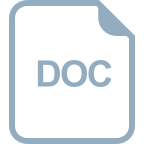
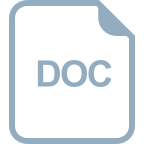
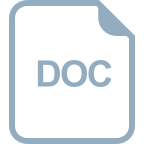
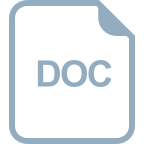
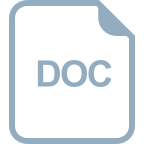
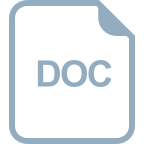
