android studio做个课程表
时间: 2023-10-09 10:11:58 浏览: 78
基于Android Studio开发的课程表APP功能非常丰富和实用。它可以获取四川大学教务处的在校学生课程表,并提供四川大学教务处的比赛信息、学院信息、讲座信息等。此外,它还具有日程表功能,可以新建、删除日程提醒。您还可以查询四川大学的课程信息。该应用还支持课程信息的添加、展示设置、上下课时间设置、字体大小设置、应用背景图片更换和授课老师的显示。您可以将设置好的课程表导出到手机内部,也可以将课程表导出到其他设备。此外,该课程表APP还具有基本的增删改查功能,界面美观,易于上手,并且支持单双周的区分,可以单独查看。
相关问题
android studio课程表
Android Studio是一款由Google开发的集成开发环境(IDE),用于开发Android应用程序。它提供了丰富的工具和功能,可以帮助开发者设计、编码、调试和测试Android应用。
关于Android Studio课程表,我不清楚你具体指的是什么内容。如果你是指学习Android Studio的课程表,通常会包括以下内容:
1. 环境搭建:学习如何下载和安装Android Studio,并配置相关的开发环境。
2. 基础知识:学习Android应用程序的基本结构、组件和生命周期,以及Java编程语言的基础知识。
3. 用户界面设计:学习如何使用Android Studio的布局编辑器创建用户界面,包括使用XML和Java代码定义布局和控件。
4. 数据存储:学习如何使用Android提供的各种数据存储方式,如SharedPreferences、SQLite数据库和文件系统。
5. 网络通信:学习如何使用Android提供的API进行网络通信,包括HTTP请求、JSON解析和图片加载等。
6. 多媒体处理:学习如何在Android应用中处理音频、视频和图像等多媒体资源。
7. 性能优化:学习如何优化Android应用的性能,包括内存管理、线程管理和网络请求优化等。
8. 调试和测试:学习如何使用Android Studio的调试工具和单元测试框架进行应用程序的调试和测试。
以上是一些常见的Android Studio课程内容,具体的课程表可能会根据不同的培训机构或学校而有所不同。
用 Android studio写一个课程表
好的,我可以给你提供一个简单的课程表的实现思路。你可以根据自己的需求进行修改和完善。
首先,我们需要创建一个主界面,用来显示整个课程表。可以使用一个GridLayout来显示课程表格子。
然后,我们需要设计数据结构来存储课程信息。一个课程需要包含课程名称、上课时间、上课地点等信息。可以设计一个Course类来存储这些信息。
接下来,我们需要实现一个课程表的适配器,用来将课程信息显示在GridLayout上。适配器需要继承自BaseAdapter,实现getView()方法来显示每个课程格子。可以在getView()方法中设置课程格子的背景颜色、课程名称和上课时间等信息。
最后,在MainActivity中,我们需要读取课程信息并且将其传递给适配器,让适配器将课程信息显示在GridLayout上。可以在onCreate()方法中读取课程信息,然后将其传递给适配器。
以上就是一个简单的课程表实现思路,你可以根据自己的需求进行修改和完善。
相关推荐
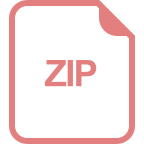
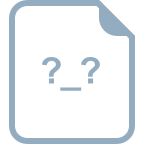












