数据结构课程设计c++图书管
时间: 2024-12-29 07:24:01 浏览: 3
### C++ 数据结构 课程设计 图书管理系统 实现方案
#### 构造函数与析构函数的作用
程序启动时,构造函数负责初始化工作,读取文件并将数据加载至内存中。具体而言,在`Library`类的构造函数内实现了对图书信息和学生信息的读取并将其保存于相应的容器中[^3]。
```cpp
class Library {
private:
vector<Book> bookshelf;
vector<Student> studentList;
public:
Library();
~Library();
};
```
#### 主要功能模块的设计思路
为了构建完整的图书管理系统,需定义多个核心组件及其交互方式:
- **书籍管理**:涉及书籍增删改查等操作;
- **借阅记录维护**:处理用户的借还请求,并跟踪每本书的状态;
- **用户权限验证**:确保只有合法注册过的成员才能访问特定资源。
#### 关键代码片段展示
##### `Book` 类定义
用于表示单本藏书的信息模型。
```cpp
#include <string>
using namespace std;
struct Book {
int id;
string title;
bool available;
explicit Book(const string& dataStr);
friend ostream &operator<<(ostream &os, const Book &book);
};
// Constructor implementation outside the class definition.
Book::Book(const string& dataStr){
stringstream ss(dataStr);
ss >> id >> title >> available;
}
ostream &operator<<(ostream &os, const Book &book) {
os << "ID: " << book.id << ", Title: " << book.title
<< (book.available ? ", Available" : ", Not Available");
return os;
}
```
##### `Student` 类定义
描述一名读者的身份属性及所关联的行为方法。
```cpp
struct Student {
int sid;
string name;
list<int> borrowedBooksIDs;
explicit Student(const string& dataStr);
void borrow(int bid);
void giveBack(int bid);
friend istream &operator>>(istream &is, Student &student);
friend ostream &operator<<(ostream &os, const Student &stu);
};
void Student::borrow(int bid){ /*...*/ }
void Student::giveBack(int bid){ /*...*/ }
Student::Student(const string& dataStr){
stringstream ss(dataStr);
ss >> sid >> name;
}
istream &operator>>(istream &is, Student &student){
is >> student.sid >> student.name;
return is;
}
ostream &operator<<(ostream &os, const Student &stu){
os << "SID:" << stu.sid << "\tName:"<< stu.name ;
return os;
}
```
##### 文件IO操作封装
通过重载流运算符简化了从磁盘读写对象的过程,使得后续业务逻辑更加清晰易懂。
---
阅读全文
相关推荐




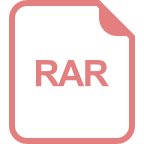




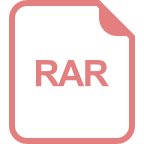



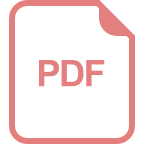



