2、先建立一个职工类Employee,包含数据成员name(姓名),ID(编号),以他为基类,派生出经理类Manager和技术人员类Technician,在经理类中增加数据成员salary(月工资),在技术人员类中增加数据成员wage(代表每小时的工资)和hours(工作时长)。在定义类时,所有类中必须包含构造函数、析构函数、修改和获取所有数据成员的函数,以及虚函数计算职工的工资、输出职工的信息。
时间: 2024-03-02 09:51:50 浏览: 103
很高兴能够回答你的问题!这是一个经典的继承与多态的例子,下面是符合你要求的职工类 Employee 及其派生类 Manager 和 Technician 的实现:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Employee {
public:
Employee(string name, int id) : name(name), id(id) {}
virtual ~Employee() {}
virtual double calculateSalary() const = 0;
virtual void printInfo() const {
cout << "Name: " << name << endl;
cout << "ID: " << id << endl;
}
void setName(string name) { this->name = name; }
string getName() const { return name; }
void setId(int id) { this->id = id; }
int getId() const { return id; }
protected:
string name;
int id;
};
class Manager : public Employee {
public:
Manager(string name, int id, double salary)
: Employee(name, id), salary(salary) {}
double calculateSalary() const {
return salary;
}
void printInfo() const {
Employee::printInfo();
cout << "Salary: " << salary << endl;
}
void setSalary(double salary) { this->salary = salary; }
double getSalary() const { return salary; }
private:
double salary;
};
class Technician : public Employee {
public:
Technician(string name, int id, double wage, int hours)
: Employee(name, id), wage(wage), hours(hours) {}
double calculateSalary() const {
return wage * hours;
}
void printInfo() const {
Employee::printInfo();
cout << "Wage: " << wage << endl;
cout << "Hours: " << hours << endl;
}
void setWage(double wage) { this->wage = wage; }
double getWage() const { return wage; }
void setHours(int hours) { this->hours = hours; }
int getHours() const { return hours; }
private:
double wage;
int hours;
};
```
在上面的代码中,我们首先定义了基类 Employee,其中包含了姓名和编号两个数据成员,以及计算工资和输出职工信息的虚函数。接着我们定义了经理类 Manager 和技术人员类 Technician,它们分别从基类 Employee 派生而来,具有自己的数据成员和计算工资/输出信息的函数。在代码中,我们使用了纯虚函数 calculateSalary(),它强制子类实现计算工资的方法,这是一种常见的实现多态性的方式。
如果你有任何问题,请继续提问!
阅读全文
相关推荐
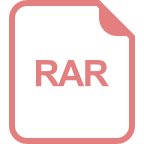
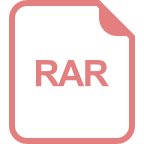







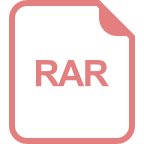
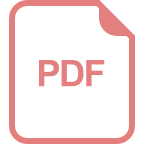
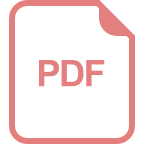
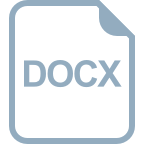
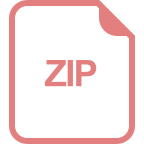
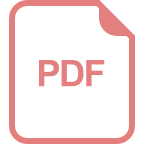
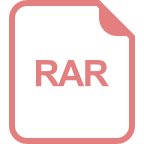
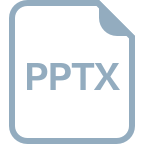