使用c++语言模拟超市购物的简单计算机系统
时间: 2024-03-18 18:41:33 浏览: 14
好的,我可以帮你解答这个问题。
首先,我们可以考虑使用面向对象的方法来设计这个系统。超市可以看作是一个类,商品可以看作是另一个类,购物车可以看作是第三个类。购物车类中包含了一个商品类的数组,用来存储购物车中的商品信息。
下面是一个简单的实现:
```c++
#include <iostream>
#include <string>
using namespace std;
class Item {
public:
string name;
double price;
Item(string n, double p) {
name = n;
price = p;
}
};
class Cart {
public:
Item* items[100];
int num_items;
Cart() {
num_items = 0;
}
void add_item(Item* item) {
items[num_items] = item;
num_items++;
}
double get_total() {
double total = 0;
for (int i = 0; i < num_items; i++) {
total += items[i]->price;
}
return total;
}
};
class Market {
public:
Item* items[100];
int num_items;
Market() {
num_items = 0;
}
void add_item(Item* item) {
items[num_items] = item;
num_items++;
}
void display_items() {
for (int i = 0; i < num_items; i++) {
cout << items[i]->name << " - " << items[i]->price << endl;
}
}
};
int main() {
// create a market
Market market;
// add some items to the market
market.add_item(new Item("apple", 1.0));
market.add_item(new Item("banana", 1.5));
market.add_item(new Item("orange", 2.0));
// display the items in the market
market.display_items();
// create a cart
Cart cart;
// add some items to the cart
cart.add_item(market.items[0]);
cart.add_item(market.items[1]);
// display the total price of the items in the cart
cout << "Total price: " << cart.get_total() << endl;
return 0;
}
```
在这个实现中,我们定义了三个类:`Item`,`Cart`和`Market`。`Item`类表示商品,包含了商品的名称和价格。`Cart`类表示购物车,包含了一个商品类的数组,用来存储购物车中的商品信息。`Market`类表示超市,包含了一个商品类的数组,用来存储超市中的商品信息。
在`main`函数中,我们首先创建了一个超市,并向其中添加了一些商品。然后,我们创建了一个购物车,并向其中添加了一些商品。最后,我们计算了购物车中商品的总价,并输出了结果。
这只是一个简单的例子,你可以根据需要进行修改和扩展。
相关推荐
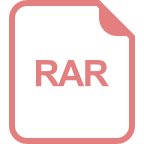
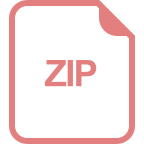














