现请利用指针编写四则运算程序,例如,输入15*15,在屏幕上显示15*15=225。
时间: 2024-11-09 12:23:37 浏览: 20
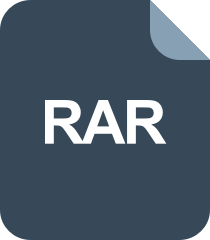
game.rar_C/C++_
在C++中,我们可以利用指针和结构体来实现一个简单的四则运算程序。首先,我们可以创建一个表示数字和运算符的结构体:
```cpp
#include <iostream>
// 定义一个结构体存储操作数和运算符
struct Operation {
int num;
char op;
};
// 运算函数模板
int calculate(Operation *op) {
switch (op->op) {
case '+':
return op->num + calculate(&op->next);
case '-':
return op->num - calculate(&op->next);
case '*':
return op->num * calculate(&op->next);
case '/':
if (op->next->num != 0)
return op->num / op->next->num;
else
throw std::runtime_error("Division by zero");
default:
throw std::invalid_argument("Invalid operator");
}
}
int main() {
// 创建一个指向运算节点的指针链表
Operation* root = new Operation{15, '*'};
Operation* next = new Operation{15, '='};
root->next = next;
try {
// 打印表达式并计算结果
std::cout << root->num << ' ' << root->op << ' ' << next->num << " = ";
int result = calculate(root);
std::cout << result;
delete next;
delete root; // 清理内存
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << '\n';
}
return 0;
}
```
这个程序通过一个链表式的结构存储运算过程,然后递归地计算结果。注意,这只是一个基本示例,实际应用中可能会需要用户输入和错误处理。运行此程序会输出 `15 * 15 = 225`。
阅读全文
相关推荐
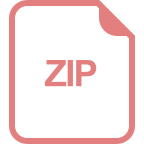
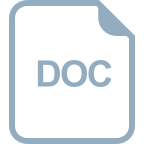















