编写一个函数将数据转化为树形结构并插入到路由中
时间: 2024-01-22 14:17:22 浏览: 289
假设输入的数据格式为:
```
[
{ "id": 1, "name": "node1", "parent_id": null },
{ "id": 2, "name": "node2", "parent_id": 1 },
{ "id": 3, "name": "node3", "parent_id": 2 },
{ "id": 4, "name": "node4", "parent_id": 1 },
{ "id": 5, "name": "node5", "parent_id": null }
]
```
其中,每个节点有一个唯一的 `id`、一个名称 `name` 和一个可选的父节点 `parent_id`。如果 `parent_id` 为 `null`,则表示该节点是根节点。
我们可以编写如下的函数将数据转化为树形结构并插入到路由中:
```javascript
function insertTreeToRouter(data, router) {
// 用一个对象来存储每个节点的信息
const nodes = {};
// 遍历数据,将每个节点加入到 nodes 对象中
data.forEach(node => {
nodes[node.id] = {
id: node.id,
name: node.name,
children: []
};
});
// 遍历数据,将每个节点加入到其父节点的 children 数组中
data.forEach(node => {
if (node.parent_id !== null) {
nodes[node.parent_id].children.push(nodes[node.id]);
}
});
// 将根节点加入到路由中
Object.values(nodes).forEach(node => {
if (node.parent_id === null) {
router.use(node.name, createRouter(node.children));
}
});
}
```
这个函数首先创建一个空对象 `nodes`,用于存储每个节点的信息。然后遍历数据,将每个节点加入到 `nodes` 对象中。接着遍历数据,将每个节点加入到其父节点的 `children` 数组中。最后,将根节点加入到路由中。
我们可以使用 `express` 框架的 `Router` 类来创建子路由,因此还需要编写一个 `createRouter` 函数来创建子路由:
```javascript
function createRouter(children) {
const router = express.Router();
// 将子节点加入到子路由中
children.forEach(child => {
router.use(child.name, createRouter(child.children));
});
return router;
}
```
这个函数首先创建一个空的子路由 `router`。然后遍历子节点,将每个子节点加入到子路由中。最后返回子路由。
现在我们可以调用 `insertTreeToRouter` 函数来将数据转化为树形结构并插入到路由中:
```javascript
const express = require('express');
const app = express();
const data = [
{ "id": 1, "name": "node1", "parent_id": null },
{ "id": 2, "name": "node2", "parent_id": 1 },
{ "id": 3, "name": "node3", "parent_id": 2 },
{ "id": 4, "name": "node4", "parent_id": 1 },
{ "id": 5, "name": "node5", "parent_id": null }
];
insertTreeToRouter(data, app);
app.listen(3000, () => {
console.log('Server started on port 3000');
});
```
这个例子中,我们将根路由 `app` 作为参数传入 `insertTreeToRouter` 函数,并将数据作为另一个参数传入。最后启动服务器并监听端口 3000。
阅读全文
相关推荐
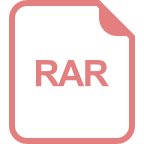


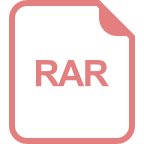
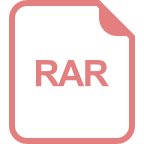

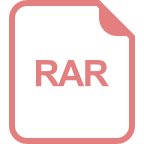
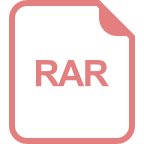
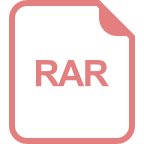








