java调用高德地图api
时间: 2023-10-02 12:06:29 浏览: 82
要在Java中调用高德地图API,你可以按照以下步骤进行操作:
1. 创建一个工具类MapUtil,并导入所需的包,例如net.sf.json、org.apache.commons.collections等。
2. 在工具类中定义你在高德官网获取的key,例如private static String key = "xxxxxxxxxxxxxxxxxxxxxxxxx";
3. 在工具类中编写方法来调用高德地图API。你可以使用Java的URL和HttpURLConnection类来发送HTTP请求,并通过API的URL和参数生成请求URL。
4. 发送HTTP请求并获取响应。可以使用URLConnection的getInputStream()方法来获取API的响应数据。
5. 解析API的响应数据。根据API文档中的返回数据格式,你可以使用JSON库解析响应数据,例如使用JSONObject和JSONArray类来解析JSON格式的数据。
6. 处理解析后的数据。你可以根据需要提取所需的信息,例如获取地理坐标、距离、路线等。
下面是一个示例代码来调用高德地图API:
```java
import net.sf.json.JSONArray;
import net.sf.json.JSONObject;
import org.apache.commons.collections.MapUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.*;
import java.net.HttpURLConnection;
import java.net.URL;
import java.net.URLConnection;
import java.util.List;
import java.util.Map;
import java.util.regex.Pattern;
public class MapUtil {
private static final Logger LOGGER = LoggerFactory.getLogger(MapUtil.class);
private static String key = "xxxxxxxxxxxxxxxxxxxxxxxxx";
public static String getResponse(String urlString) throws IOException {
StringBuilder result = new StringBuilder();
URL url = new URL(urlString);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
connection.setConnectTimeout(5000);
connection.setReadTimeout(5000);
BufferedReader reader = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String line;
while ((line = reader.readLine()) != null) {
result.append(line);
}
reader.close();
connection.disconnect();
return result.toString();
}
public static JSONObject getJson(String urlString) throws IOException {
String response = getResponse(urlString);
return JSONObject.fromObject(response);
}
public static void main(String[] args) {
try {
String apiUrl = "https://restapi.amap.com/v3/place/text?keywords=北京&key=" + key;
JSONObject result = getJson(apiUrl);
// 解析结果
JSONArray pois = result.getJSONArray("pois");
for (int i = 0; i < pois.size(); i++) {
JSONObject poi = pois.getJSONObject(i);
String name = poi.getString("name");
String location = poi.getString("location");
System.out.println(name + ": " + location);
}
} catch (IOException e) {
LOGGER.error("Failed to call AMap API: " + e.getMessage());
}
}
}
```
请注意,这只是一个示例代码,你可以根据具体需求进行修改和扩展。你可以根据高德地图API文档提供的接口和参数来调用不同的功能和服务。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* *3* [SpringCloud之如何在项目中调用高德地图API](https://blog.csdn.net/qq_31122833/article/details/80653419)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 100%"]
[ .reference_list ]
相关推荐
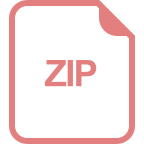














