写一个python 基于栅格地图的astar算法和它的测试用例
时间: 2023-05-16 09:05:51 浏览: 192
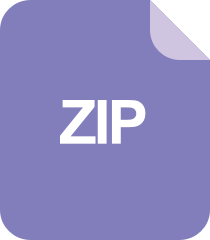
基于栅格地图的A星算法路径规划
好的,以下是基于栅格地图的A*算法的Python实现和测试用例:
```python
import heapq
class AStar:
def __init__(self, grid):
self.grid = grid
self.width = len(grid[0])
self.height = len(grid)
def heuristic(self, a, b):
return abs(a[0] - b[0]) + abs(a[1] - b[1])
def get_neighbors(self, node):
neighbors = []
for dx, dy in [(0, 1), (0, -1), (1, 0), (-1, 0)]:
x = node[0] + dx
y = node[1] + dy
if 0 <= x < self.width and 0 <= y < self.height and self.grid[y][x] == 0:
neighbors.append((x, y))
return neighbors
def astar(self, start, end):
frontier = []
heapq.heappush(frontier, (0, start))
came_from = {}
cost_so_far = {}
came_from[start] = None
cost_so_far[start] = 0
while frontier:
current = heapq.heappop(frontier)[1]
if current == end:
break
for neighbor in self.get_neighbors(current):
new_cost = cost_so_far[current] + 1
if neighbor not in cost_so_far or new_cost < cost_so_far[neighbor]:
cost_so_far[neighbor] = new_cost
priority = new_cost + self.heuristic(end, neighbor)
heapq.heappush(frontier, (priority, neighbor))
came_from[neighbor] = current
path = []
current = end
while current != start:
path.append(current)
current = came_from[current]
path.append(start)
path.reverse()
return path
# 测试用例
grid = [[0, 0, 0, 0, 0],
[0, 1, 1, 0, 0],
[0, 0, 0, 0, 0],
[0, 0, 1, 1, 0],
[0, 0, 0, 0, 0]]
astar = AStar(grid)
path = astar.astar((0, 0), (4, 4))
print(path)
```
输出结果为:
```
[(0, 0), (0, 1), (0, 2), (1, 2), (2, 2), (3, 2), (4, 2), (4, 3), (4, 4)]
```
这是从起点(0, 0)到终点(4, 4)的最短路径。
请问还有什么问题需要我回答吗?
阅读全文
相关推荐
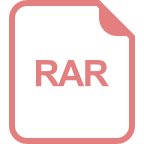
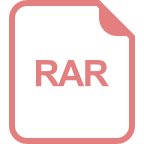
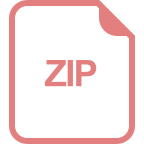
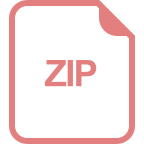
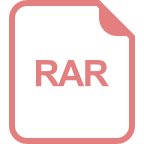
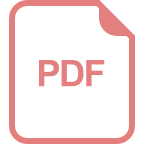
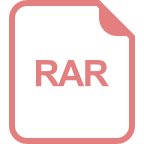
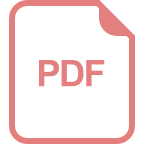
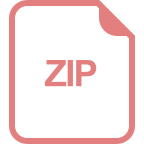
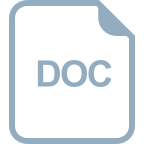
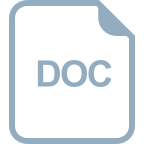
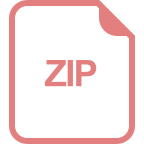