controller返回json
时间: 2023-06-30 21:18:39 浏览: 49
在 Web 应用程序中,通常使用 Spring MVC 或 Spring Boot 等框架来开发 RESTful API,以提供 JSON 格式的数据。在 Spring MVC 中,可以使用 `@RestController` 注解来标记一个类,将其作为 RESTful API 的控制器,然后使用 `@RequestMapping` 或其他注解来定义不同的请求路径和 HTTP 方法。
以下是一个简单的示例,演示如何使用 Spring MVC 返回 JSON 数据:
```java
@RestController
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/users")
public List<User> getUsers() {
return userService.getUsers();
}
@GetMapping("/users/{id}")
public User getUserById(@PathVariable Long id) {
return userService.getUserById(id);
}
}
```
在这个示例中,`UserController` 类被标记为 `@RestController`,表示它是一个 RESTful API 的控制器。`userService` 是一个用户服务类的实例,它被自动注入到控制器中。`getUsers` 方法使用 `@GetMapping("/users")` 注解来定义了 `/users` 路径和 HTTP GET 方法,它将返回一个包含所有用户的 JSON 数组。`getUserById` 方法使用 `@GetMapping("/users/{id}")` 注解来定义了带有路径变量的路径和 HTTP GET 方法,它将返回具有指定 ID 的用户的 JSON 对象。
当客户端发送 GET 请求到 `/users` 路径时,服务器将返回一个 JSON 数组,其中包含所有用户的信息。例如:
```json
[
{
"id": 1,
"name": "Alice",
"age": 25
},
{
"id": 2,
"name": "Bob",
"age": 30
},
{
"id": 3,
"name": "Charlie",
"age": 35
}
]
```
当客户端发送 GET 请求到 `/users/1` 路径时,服务器将返回一个具有 ID 为 1 的用户的 JSON 对象。例如:
```json
{
"id": 1,
"name": "Alice",
"age": 25
}
```
以上示例中使用了 Spring Boot,但是同样的代码也适用于 Spring MVC。
相关推荐
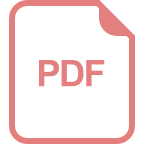
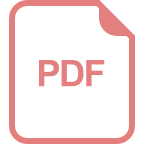














