使用python构建可以发送信息、图片、文件,并且附带GUI界面的聊天系统。
时间: 2024-02-04 21:04:19 浏览: 166
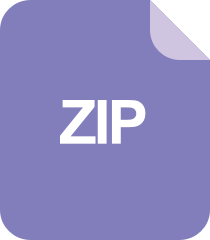
python制作电子词典、GUI界面
这个问题需要分几个步骤来实现:
1. 使用Python的socket模块创建一个服务器和客户端之间的连接,以便在两者之间传输数据。
2. 使用Python的tkinter模块创建GUI界面,并添加聊天窗口、输入框和发送按钮等组件。
3. 在Python中编写代码,以便在聊天窗口中显示已接收到的消息,并将发送按钮与发送消息的功能绑定。
4. 通过Python的Pillow模块使程序能够发送图片文件,通过Python的os模块使程序能够发送文本文件。
下面是一个简单的示例代码,可以作为起点来构建更复杂的聊天系统:
```python
import socket
import threading
import os
from tkinter import *
from tkinter import filedialog
from PIL import ImageTk, Image
# 创建socket连接
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.connect(('localhost', 9999))
# 创建GUI界面
root = Tk()
root.title("聊天系统")
root.geometry("500x500")
# 创建聊天窗口
chat_window = Text(root, bd=1, bg="white", height="8", width="50", font="Arial")
chat_window.place(x=6, y=6, height=386, width=370)
# 创建输入框
message_entry = Entry(root, bd=0, bg="white", font="Arial")
message_entry.place(x=128, y=400, height=88, width=260)
# 创建发送按钮
send_button = Button(root, text="发送", font="Arial", width="10", height=5, bd=0, bg="#f9a602", activebackground="#3c9d9b", fg='#000000', command=lambda: send_message())
send_button.place(x=6, y=400, height=88)
# 创建文件选择按钮
file_button = Button(root, text="选择文件", font="Arial", width="10", height=2, bd=0, bg="#f9a602", activebackground="#3c9d9b", fg='#000000', command=lambda: open_file())
file_button.place(x=380, y=400, height=30)
# 创建图片选择按钮
image_button = Button(root, text="选择图片", font="Arial", width="10", height=2, bd=0, bg="#f9a602", activebackground="#3c9d9b", fg='#000000', command=lambda: open_image())
image_button.place(x=380, y=450, height=30)
# 打开文件选择对话框,选择要发送的文件,并将其发送到服务器
def open_file():
file_path = filedialog.askopenfilename()
if file_path != '':
file_name = os.path.basename(file_path)
file_size = os.path.getsize(file_path)
s.send(("file|" + file_name + "|" + str(file_size)).encode())
with open(file_path, 'rb') as f:
s.sendall(f.read())
# 打开图片选择对话框,选择要发送的图片,并将其发送到服务器
def open_image():
image_path = filedialog.askopenfilename()
if image_path != '':
image = Image.open(image_path)
image = image.resize((100, 100), Image.ANTIALIAS)
photo = ImageTk.PhotoImage(image)
chat_window.image_create(END, image=photo)
chat_window.insert(END, "\n")
s.send(("image|" + image_path).encode())
# 接收来自服务器的消息,并将其显示在聊天窗口中
def receive_message():
while True:
message = s.recv(1024).decode()
if message.startswith('file|'):
_, file_name, file_size = message.split("|")
file_size = int(file_size)
with open(file_name, 'wb') as f:
bytes_read = 0
while bytes_read < file_size:
data = s.recv(1024)
f.write(data)
bytes_read += len(data)
chat_window.insert(END, "文件 " + file_name + " 发送成功\n")
elif message.startswith('image|'):
_, image_path = message.split("|")
chat_window.insert(END, "图片 " + image_path + " 发送成功\n")
else:
chat_window.insert(END, message + "\n")
# 将输入框中的消息发送给服务器,并将其显示在聊天窗口中
def send_message():
message = message_entry.get()
s.send(message.encode())
chat_window.insert(END, "你: " + message + "\n")
message_entry.delete(0, END)
# 启动一个线程来接收来自服务器的消息
receive_thread = threading.Thread(target=receive_message)
receive_thread.start()
# 启动GUI界面
root.mainloop()
```
注意:以上代码仅作为示例,需要根据实际情况进行修改。此外,还需要在服务器端编写代码,以便接收来自客户端的消息和文件,并将其转发给其他客户端。
阅读全文
相关推荐
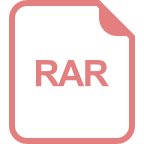
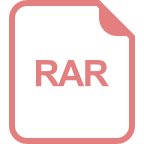















