#include <iostream> #include <cstring> using namespace std; bool is_palindrome(string s) { int n = s.length(); for (int i = 0; i < n / 2; i++) { if (s[i] != s[n - i - 1]) { return false; } } return true; } int main() { int n; cin >> n; cin.ignore(); // 忽略输入中的换行符 for (int i = 0; i < n; i++) { string s; getline(cin, s); if (is_palindrome(s)) { cout << "The string is a palindrome" << endl; } else { cout << "The string is not a palindrome" << endl; } } return 0; }设置为自定义的十个字符串
时间: 2024-01-11 16:03:56 浏览: 66
可以将程序中的 `n` 改为 10,然后修改循环体中的输入语句,如下所示:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
bool is_palindrome(string s) {
int n = s.length();
for (int i = 0; i < n / 2; i++) {
if (s[i] != s[n - i - 1]) {
return false;
}
}
return true;
}
int main() {
const int n = 10;
string strs[n] = {"abccba", "1234321", "abcba", "123321", "racecar", "hello", "world", "madam", "level", "deified"};
for (int i = 0; i < n; i++) {
if (is_palindrome(strs[i])) {
cout << "The string is a palindrome" << endl;
} else {
cout << "The string is not a palindrome" << endl;
}
}
return 0;
}
```
这个程序中我们定义了一个字符串数组 `strs`,其中包含了 10 个字符串。在循环体中,我们遍历 `strs` 数组中的每个字符串,调用 `is_palindrome` 函数来判断每个字符串是否是回文字符串,并输出相应的信息。
阅读全文
相关推荐
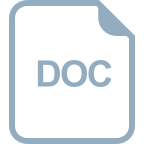
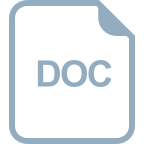
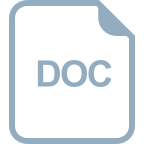


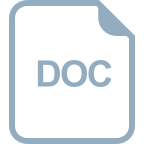
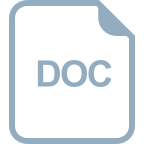
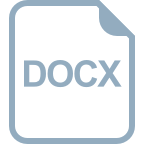
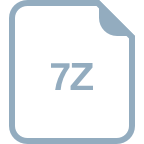
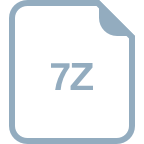
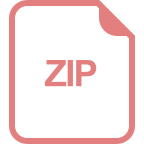
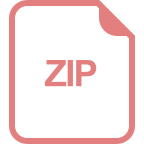
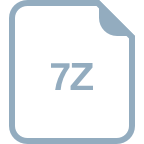
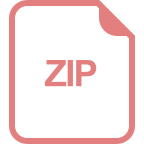