python如何将多次结果存入不同文件夹
时间: 2024-05-09 18:19:46 浏览: 15
你可以使用Python的os模块来创建不同的文件夹,并将结果存储在这些文件夹中。以下是一个示例代码:
```python
import os
# 创建文件夹
for i in range(3):
folder_path = f"results_{i}"
if not os.path.exists(folder_path):
os.makedirs(folder_path)
# 保存结果至不同的文件夹
for i in range(3):
folder_path = f"results_{i}"
file_path = os.path.join(folder_path, f"result_{i}.txt")
with open(file_path, "w") as f:
f.write(f"Result {i}")
```
在上面的代码中,我们首先使用一个循环来创建三个文件夹(results_0,results_1和results_2)。然后,我们使用第二个循环将结果存储在这些文件夹中。每个结果将保存在名称为result_i.txt的文件中,其中i是结果的索引。这些文件将存储在与其索引相匹配的文件夹中。
相关问题
python将文件存入另一文件夹下
可以使用shutil库中的move()函数将文件从原文件夹移动到目标文件夹。具体代码如下:
import shutil
src_file = 'path/to/source/file'
dst_folder = 'path/to/destination/folder'
shutil.move(src_file, dst_folder)
python将多个文件夹重命名为不同的名字
如果你想将多个文件夹重命名为不同的名字,可以在代码中使用字典来存储每个文件夹的原名和新名字,然后遍历字典进行重命名操作。以下是示例代码:
```python
import os
# 指定要重命名的文件夹所在的目录
folder_path = '/path/to/folder'
# 定义一个字典,存储每个文件夹的原名和新名字
folder_names = {
'old_folder_name_1': 'new_folder_name_1',
'old_folder_name_2': 'new_folder_name_2',
'old_folder_name_3': 'new_folder_name_3',
}
# 遍历字典,对每个文件夹进行重命名
for old_name, new_name in folder_names.items():
old_path = os.path.join(folder_path, old_name)
new_path = os.path.join(folder_path, new_name)
os.rename(old_path, new_path)
```
在上面的示例代码中,我们定义了一个字典`folder_names`,用来存储每个文件夹的原名和新名字。然后,使用`items()`方法遍历字典,并使用`os.path.join()`函数将文件夹名字和路径拼接起来,最后使用`os.rename()`函数进行重命名操作。
你可以根据自己的需求修改代码中的文件夹名字和路径来实现重命名操作。
相关推荐
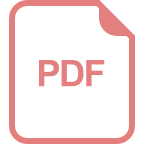
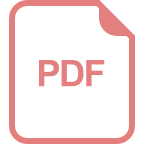
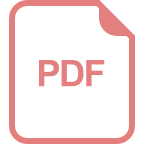
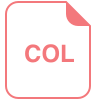
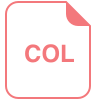
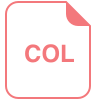
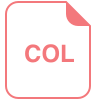
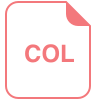







