vue3-ace-editor 封装并添加自定义语言 示例
时间: 2023-10-29 16:02:14 浏览: 631
1. 安装 vue3-ace-editor
```shell
npm install vue3-ace-editor
```
2. 在需要使用的组件中引入 ace-editor 组件
```html
<template>
<AceEditor
v-model="code"
:options="options"
mode="myCustomLanguage"
:highlightActiveLine="true"
:tabSize="2"
theme="twilight"
style="height: 500px;"
/>
</template>
<script>
import AceEditor from "vue3-ace-editor";
export default {
components: {
AceEditor,
},
data() {
return {
code: "",
options: {
enableBasicAutocompletion: true,
enableSnippets: true,
enableLiveAutocompletion: true,
},
};
},
mounted() {
this.$nextTick(() => {
this.code = "function myFunction() {\n console.log('Hello World!');\n}";
});
},
};
</script>
```
3. 添加自定义语言
```javascript
import ace from "ace-builds";
import "ace-builds/src-noconflict/mode-javascript"; // 引入 JavaScript 语言模块
ace.define(
"ace/mode/myCustomLanguage_highlight_rules",
function (require, exports) {
var oop = require("ace/lib/oop");
var TextHighlightRules = require("ace/mode/text_highlight_rules")
.TextHighlightRules;
var MyCustomLanguageHighlightRules = function () {
// 注释
this.$rules["start"] = [
{
token: "comment",
regex: "//.*$",
},
];
// 关键字
this.$rules["start"].push({
token: "keyword",
regex: "function",
});
// 函数名
this.$rules["start"].push({
token: "entity.name.function",
regex: "myFunction",
});
// 字符串
this.$rules["start"].push({
token: "string",
regex: "'|\"",
next: "string",
});
// 其他
this.$rules["start"].push({
defaultToken: "text",
});
this.$rules.string = [
{
token: "constant.language.escape",
regex: /\\(?:['"\\]|[0-7]{3})/,
},
{
token: "string",
regex: /['"]/,
next: "start",
},
{
defaultToken: "string",
},
];
};
oop.inherits(MyCustomLanguageHighlightRules, TextHighlightRules);
exports.MyCustomLanguageHighlightRules = MyCustomLanguageHighlightRules;
}
);
ace.define("ace/mode/myCustomLanguage", function (require, exports) {
var oop = require("ace/lib/oop");
var TextMode = require("ace/mode/text").Mode;
var MyCustomLanguageHighlightRules = require("./myCustomLanguage_highlight_rules")
.MyCustomLanguageHighlightRules;
var MyCustomLanguageMode = function () {
this.HighlightRules = MyCustomLanguageHighlightRules;
};
oop.inherits(MyCustomLanguageMode, TextMode);
(function () {
this.$id = "ace/mode/myCustomLanguage";
}).call(MyCustomLanguageMode.prototype);
exports.Mode = MyCustomLanguageMode;
});
```
4. 在组件中引入自定义语言模块
```javascript
import "ace-builds/src-noconflict/mode-myCustomLanguage"; // 引入自定义语言模块
```
5. 完整代码
```html
<template>
<AceEditor
v-model="code"
:options="options"
mode="myCustomLanguage"
:highlightActiveLine="true"
:tabSize="2"
theme="twilight"
style="height: 500px;"
/>
</template>
<script>
import AceEditor from "vue3-ace-editor";
import "ace-builds/src-noconflict/mode-myCustomLanguage"; // 引入自定义语言模块
export default {
components: {
AceEditor,
},
data() {
return {
code: "",
options: {
enableBasicAutocompletion: true,
enableSnippets: true,
enableLiveAutocompletion: true,
},
};
},
mounted() {
this.$nextTick(() => {
this.code = "function myFunction() {\n console.log('Hello World!');\n}";
});
},
};
</script>
```
相关推荐
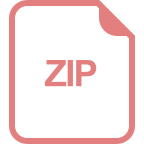














