selenium自动化测试鼠标移动滚轮
时间: 2023-07-12 18:05:16 浏览: 152
要在Selenium中模拟鼠标移动滚轮的操作,可以使用ActionChains类的move_by_offset()和perform()方法。
以下是一个示例代码:
```python
from selenium import webdriver
from selenium.webdriver.common.action_chains import ActionChains
driver = webdriver.Chrome()
# 打开网页
driver.get("https://www.example.com")
# 创建ActionChains对象
actions = ActionChains(driver)
# 将鼠标移动至某个元素
element = driver.find_element_by_id("example-element")
actions.move_to_element(element)
# 使用move_by_offset()方法模拟鼠标滚轮滚动
actions.move_by_offset(0, 500).perform()
# 关闭浏览器
driver.quit()
```
在这个示例中,我们首先创建了一个ActionChains对象,并使用move_to_element()方法将鼠标移动到页面中的某个元素上。接下来,我们使用move_by_offset()方法来模拟鼠标滚轮向下滚动500个像素的操作。最后,我们使用perform()方法来执行这个动作。
相关问题
python selenium自动化测试58同城滑动浏览功能
58同城网站实现滑动浏览的方式是通过模拟鼠标滚轮滑动来实现的,因此我们可以使用Selenium中的ActionChains类来模拟这个动作。具体步骤如下:
1. 导入selenium和ActionChains类。
```python
from selenium import webdriver
from selenium.webdriver.common.action_chains import ActionChains
```
2. 启动浏览器并访问58同城网站。
```python
driver = webdriver.Chrome()
driver.get("https://www.58.com/")
```
3. 定位到需要滑动的元素。
```python
ele = driver.find_element_by_xpath("//div[@class='house-list-wrap']")
```
4. 使用ActionChains类模拟鼠标滚轮滑动。
```python
actions = ActionChains(driver)
actions.move_to_element(ele).perform()
for i in range(5):
actions.move_by_offset(0, 500).perform()
time.sleep(1)
```
以上代码中,我们首先使用move_to_element()方法将鼠标移动到需要滑动的元素上,然后使用move_by_offset()方法模拟鼠标滚轮滑动,并使用time.sleep()方法等待网页加载完成。
完整代码如下:
```python
import time
from selenium import webdriver
from selenium.webdriver.common.action_chains import ActionChains
driver = webdriver.Chrome()
driver.get("https://www.58.com/")
ele = driver.find_element_by_xpath("//div[@class='house-list-wrap']")
actions = ActionChains(driver)
actions.move_to_element(ele).perform()
for i in range(5):
actions.move_by_offset(0, 500).perform()
time.sleep(1)
driver.quit()
```
希望这个例子能够帮助你实现58同城自动化测试中的滑动浏览功能。
selenium 只对某个区域内鼠标滚轮操作
### 回答1:
可以使用 `ActionChains` 类中的 `move_to_element` 方法来将鼠标移动到需要操作的区域内,然后再使用 `send_keys` 方法模拟滚轮操作。示例代码如下:
```python
from selenium import webdriver
from selenium.webdriver.common.action_chains import ActionChains
driver = webdriver.Chrome()
driver.get('https://www.example.com')
# 定位需要操作的区域
element = driver.find_element_by_id('some-id')
# 将鼠标移动到该区域内
actions = ActionChains(driver)
actions.move_to_element(element)
# 模拟鼠标滚轮操作
actions.send_keys(Keys.PAGE_DOWN).perform()
```
在上述示例代码中,我使用了 `move_to_element` 方法将鼠标移动到 ID 为 `some-id` 的元素内,然后使用 `send_keys` 方法模拟了鼠标向下滚动一页的操作。你可以根据需要修改代码以实现其他滚动方式。
### 回答2:
Selenium是一种自动化测试工具,用于模拟用户在浏览器中进行各种操作。对于鼠标滚轮操作,Selenium可以调用相关的API来模拟滚动操作。
如果想要只对某个区域内执行鼠标滚轮操作,可以借助Selenium的ActionChains类来实现。ActionChains类提供了许多与鼠标操作相关的方法,包括滚动操作。
首先,我们需要定位到要操作的元素或区域。可以使用Selenium提供的定位元素的方法,如find_element_by_id、find_element_by_xpath等,来定位到要操作的元素或区域。
然后,我们可以利用ActionChains类的move_to_element_with_offset方法,将鼠标移动到要操作区域的特定位置。该方法接受两个参数,第一个参数是要移动到的元素,第二个参数是相对于该元素的偏移量。通过调整偏移量,可以精确地定位到要操作的区域。
接下来,我们可以调用ActionChains类的move_by_offset方法,传入鼠标滚动的偏移量,来模拟鼠标在该区域内进行滚动操作。通过调整滚动的偏移量,可以实现向上或向下滚动。
最后,我们可以调用perform方法来执行这一系列的操作。
综上所述,通过使用Selenium的ActionChains类,结合定位元素和鼠标滚动的偏移量,我们可以实现只对某个区域内进行鼠标滚轮操作。
### 回答3:
Selenium可以通过模拟鼠标滚轮操作来实现对一个特定区域内的滚动。首先,我们需要利用Selenium定位到需要操作的特定区域。可以使用元素的XPath、ID、类名等方式进行定位。然后,使用Selenium提供的`Actions`类来模拟鼠标操作。
具体操作步骤如下:
1. 导入Selenium库和`webdriver`模块:
```python
from selenium import webdriver
from selenium.webdriver.common.action_chains import ActionChains
```
2. 创建webdriver实例:
```python
driver = webdriver.Chrome()
```
3. 打开网页并定位到需要操作的特定区域:
```python
driver.get("网页链接")
element = driver.find_element_by_xpath("区域元素的XPath")
```
4. 实例化`ActionChains`对象,并将定位到的元素传递给它:
```python
actions = ActionChains(driver)
actions.move_to_element(element)
```
5. 根据需求进行具体的滚动操作:
```python
# 向上滚动
actions.move_by_offset(0, -200) # 按需调整偏移量
actions.perform()
# 向下滚动
actions.move_by_offset(0, 200) # 按需调整偏移量
actions.perform()
```
通过以上步骤,我们可以在特定区域内模拟鼠标滚轮的操作,并实现滚动效果。请注意,以上代码仅为示例,具体的网页结构和元素定位可能会有所不同,需要根据实际情况进行调整。
阅读全文
相关推荐
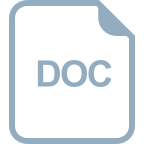
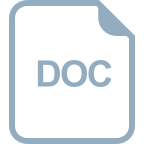
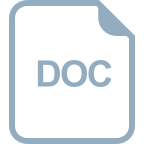
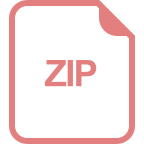
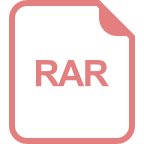
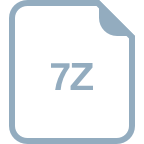
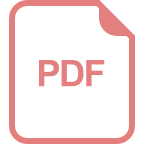
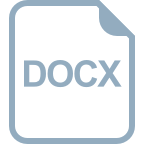
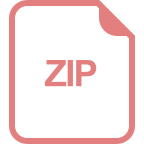
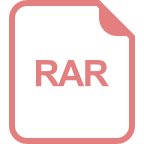
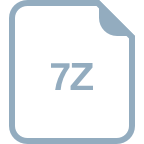
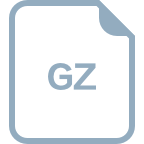
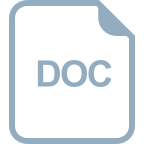
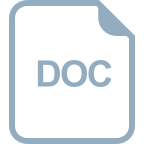
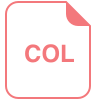
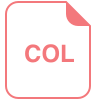
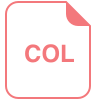