QT绘制圆角矩形边框
时间: 2024-09-26 18:00:23 浏览: 7
在Qt中,你可以使用`QPainter`类以及它的`drawRoundedRect()`函数来绘制带圆角的矩形边框。这个函数允许你在给定的位置和大小的基础上添加圆形边缘。以下是基本步骤:
1. 首先,你需要创建一个`QPainter`对象,并将其绑定到你要绘画的`QWidget`或者其他支持绘画的表面。
```cpp
QPainter painter(widget); // widget是你想要绘图的控件
```
2. 然后,你可以设置画笔的颜色和其他属性,比如宽度。
```cpp
painter.setPen(QColor("your_color")); // 设置线条颜色
painter.setBrush(Qt::NoBrush); // 或者设置填充色,这里设为无填充
painter.setRenderHint(QPainter::Antialiasing, true); // 开启抗锯齿效果,让圆角更平滑
```
3. 使用`drawRoundedRect()`函数绘制圆角矩形,传入左上角的坐标、宽高、以及圆角半径。
```cpp
int x = 0; // 左上角x坐标
int y = 0; // 左上角y坐标
int width = your_width;
int height = your_height;
int radius = your_radius; // 圆角半径
painter.drawRoundedRect(QRect(x, y, width, height), radius, radius);
```
相关问题
pyQT5绘制圆角矩形边框
在PyQt5中,要绘制带圆角的矩形边框,你可以使用`QPainter`和`QStyleOptionFrame`以及`drawRoundedRect()`函数。首先,你需要创建一个`QStyleOptionFrame`实例,然后设置相关的样式选项,最后通过`QWidget`的`paintEvent()`方法来绘制。
以下是一个简单的例子:
```python
from PyQt5.QtWidgets import QWidget, QPainter, QStyleOptionFrame, QApplication
from PyQt5.QtGui import QPainterPath, QRectF
class RoundedRectWidget(QWidget):
def __init__(self, radius=10, *args, **kwargs):
super().__init__(*args, **kwargs)
self.radius = radius
self.initUI()
def initUI(self):
self.setMouseTracking(True) # 监听鼠标移动事件
def paintEvent(self, event):
opt = QStyleOptionFrame()
opt.initFrom(self)
painter = QPainter(self)
painter.fillRect(event.rect(), Qt.lightGray) # 先填充背景颜色
painter.setPen(Qt.black) # 设置画笔颜色
path = QPainterPath(QRectF(0, 0, self.width(), self.height()))
path.addRoundedRect(event.rect(), self.radius, self.radius)
painter.drawPath(path) # 绘制圆角矩形
def mouseMoveEvent(self, event):
self.update() # 当鼠标移动时更新绘图区域
if __name__ == "__main__":
app = QApplication([])
widget = RoundedRectWidget()
widget.show()
app.exec_()
```
在这个例子中,`radius`参数控制圆角的大小。当你在窗口内移动鼠标时,你会看到随着鼠标位置的变化,圆角矩形边框会跟随移动。
QPainter 绘制透明圆角矩形 drawRoundRect
`QPainter`是Qt框架中用于2D图形绘制的一个类。通过`QPainter`对象,你可以在`QWidget`或者`QPixmap`等绘图设备上绘制各种图形。`drawRoundRect`方法是`QPainter`提供的一个绘制圆角矩形的方法。
使用`QPainter`的`drawRoundRect`方法绘制透明圆角矩形,需要结合透明度相关的知识。在Qt中,你可以通过设置画笔的填充颜色和画刷(`QBrush`)来实现透明效果。比如,你可以创建一个`QBrush`对象,其颜色设置为带有透明度的(例如,使用`Qt::white`并设置透明度),然后将其应用到`QPainter`对象上。此外,需要确保在绘制之前,窗口或绘图设备支持透明度。
以下是一个简单的例子,展示如何使用`QPainter`绘制一个具有透明效果的圆角矩形:
```cpp
void Widget::paintEvent(QPaintEvent *event) {
QPainter painter(this);
painter.setRenderHint(QPainter::Antialiasing); // 开启抗锯齿
// 创建一个半透明的画刷
QBrush brush(QColor(255, 255, 255, 127)); // 最后一个参数是透明度(0-255)
painter.setBrush(brush); // 设置画刷
painter.setPen(Qt::NoPen); // 不绘制边框
// 绘制圆角矩形
QRectF rect(50, 50, 200, 100); // 定义矩形的位置和大小
QRectF ellipse(50, 50, 200, 100); // 定义椭圆的外接矩形,用于控制圆角大小
painter.drawRoundRect(rect, 50, 50); // 绘制圆角矩形,最后两个参数控制圆角的水平和垂直半径
}
```
在上述代码中,我们首先开启抗锯齿渲染提示,然后创建了一个带有透明度的颜色作为画刷,并将其设置给`QPainter`对象。接着,我们定义了一个矩形,并使用`drawRoundRect`方法来绘制圆角矩形。这里的`ellipse`参数是一个`QRectF`对象,它定义了圆角的大小,其值为矩形宽高的一半时,可以得到较为均匀的圆角效果。
相关推荐
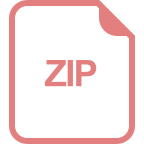
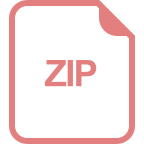
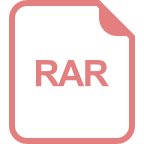













