编写一个计算三角函数值的python编程
时间: 2024-03-07 19:47:46 浏览: 124
下面是一个计算三角函数值的Python程序:
```python
import math
# 输入角度值
angle = float(input("请输入角度值:"))
# 将角度值转换为弧度值
rad = math.radians(angle)
# 计算三角函数值
sin = math.sin(rad)
cos = math.cos(rad)
tan = math.tan(rad)
cot = 1 / tan
sec = 1 / cos
csc = 1 / sin
# 输出结果
print("sin({}) = {}".format(angle, sin))
print("cos({}) = {}".format(angle, cos))
print("tan({}) = {}".format(angle, tan))
print("cot({}) = {}".format(angle, cot))
print("sec({}) = {}".format(angle, sec))
print("csc({}) = {}".format(angle, csc))
```
该程序首先要求用户输入一个角度值,然后将其转换为弧度值,并使用Python中的math库计算其对应的正弦、余弦、正切、余切、正割和余割值,最后输出结果。
注意:输入的角度值应该是一个实数,而不是一个整数。
相关问题
在编程中如何利用数学函数库实现三角函数的精确计算?请以Python为例说明。
在编程中,数学函数的使用是基础且关键的技能,特别是在科学计算和工程领域。为了解答这一问题,我推荐参考经典资料《数学函数手册(Handbook of Mathematical Functions)》,这本由Abramowitz和Stegun编写的书籍是数学函数领域的权威之作,其第十次印刷版本提供了详细的函数定义和性质,是学习和应用数学函数不可或缺的资源。
参考资源链接:[数学函数手册(Handbook of Mathematical Functions)](https://wenku.csdn.net/doc/6412b6edbe7fbd1778d4877d?spm=1055.2569.3001.10343)
在Python中,实现三角函数的精确计算通常会用到math库。math库中的函数,如sin(), cos()和tan(),提供了基本的三角函数计算。为了展示如何使用这些函数,我将提供一个简单的示例代码。请注意,这些函数默认接受弧度作为参数,如果输入的是角度,则需要将其转换为弧度。
```python
import math
# 将角度转换为弧度
def degrees_to_radians(degrees):
return degrees * math.pi / 180
# 计算三角函数值
angle_degrees = 30 # 30度角
angle_radians = degrees_to_radians(angle_degrees)
sin_value = math.sin(angle_radians)
cos_value = math.cos(angle_radians)
tan_value = math.tan(angle_radians)
print(f
参考资源链接:[数学函数手册(Handbook of Mathematical Functions)](https://wenku.csdn.net/doc/6412b6edbe7fbd1778d4877d?spm=1055.2569.3001.10343)
用python编写一个测试程序,读入三角形三边的值,若输人有效则计算面积。否则,显示输人无效计算三角形面积的公式在编程题2.14 中给出
```
# 定义函数计算三角形面积
def triangle_area(a, b, c):
if a + b > c and a + c > b and b + c > a:
p = (a + b + c) / 2
s = (p * (p - a) * (p - b) * (p - c)) ** 0.5
return s
else:
return None
# 读入三角形三边的值
a = float(input("请输入第一条边长:"))
b = float(input("请输入第二条边长:"))
c = float(input("请输入第三条边长:"))
# 计算面积并输出结果
s = triangle_area(a, b, c)
if s:
print("三角形的面积为:", s)
else:
print("输入无效,无法计算三角形面积。")
print("三角形面积公式:S = sqrt[p(p-a)(p-b)(p-c)],其中p为半周长。")
阅读全文
相关推荐
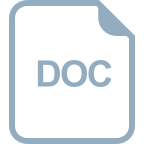
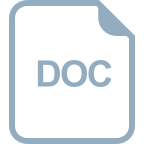
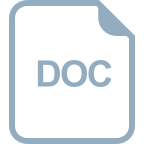
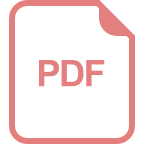
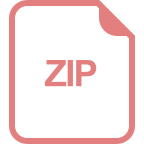
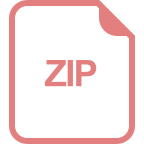
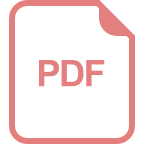
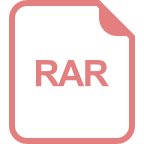
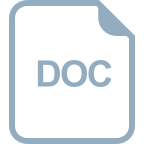
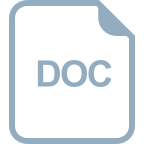
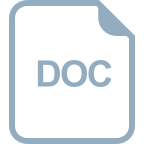
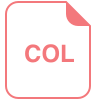




