输入n个单词,计算每个单词长度。对单词长度排序,分行输出单词长度及其单词。
时间: 2023-05-31 10:18:17 浏览: 285
### 回答1:
好的,以下是回复:
可以使用Python编程语言来实现这个功能。具体步骤如下:
1. 输入n个单词,可以使用input()函数来实现。
2. 计算每个单词长度,可以使用len()函数来实现。
3. 对单词长度排序,可以使用sorted()函数来实现。
4. 分行输出单词长度及其单词,可以使用for循环来实现。
下面是示例代码:
words = input("请输入单词,以空格分隔:").split()
word_len = [len(word) for word in words]
sorted_len = sorted(set(word_len))
for length in sorted_len:
for word in words:
if len(word) == length:
print(length, word)
希望能对您有所帮助!
### 回答2:
对于这个问题的解答,我们首先需要对输入的n个单词进行分割,并对其进行长度计算。可以使用字符串的split方法对单词进行分割,并使用len方法计算各个单词的长度,将结果存储在列表或字典中。
接下来,需要对单词长度进行排序。可以使用Python内置的sorted函数对长度进行排序。在排序时,可以使用lambda函数对每个单词的长度进行排序。
最后,需要将排序后的结果按照格式输出。可以使用for循环遍历排序后的结果,将单词长度和对应的单词按照要求输出。具体实现方式可以在每次输出时使用print函数,并使用字符串格式化或字符串拼接的方式输出。
下面是可能的代码实现:
```
words = input("请输入单词,用空格隔开:").split()
word_len_dict = {word: len(word) for word in words}
sorted_words = sorted(word_len_dict.items(), key=lambda x: x[1])
for item in sorted_words:
print("{}\t{}".format(item[1], item[0]))
```
在运行以上代码后,即可对输入的单词进行排序并输出。其中的字符串格式化方式可以根据实际情况进行调整,以满足题目要求。
### 回答3:
该问题可以用Python语言进行解决。
首先,我们需要用input()函数来获取用户输入的单词。我们可以使用split()函数来将输入的所有单词分割成一个单独的字符串列表。然后,我们可以使用len()函数来计算每个单词的长度,并将其存储在一个新的列表中。
```
words = input("请输入单词,以空格分隔: ").split()
word_len = [len(word) for word in words]
```
接下来,我们需要对单词长度进行排序并打印输出。我们可以使用Python的sorted()函数来对列表进行排序,并将其设置为基于长度排序。然后,我们可以使用zip()函数来将排序后的单词长度和单词列表进行配对,从而将它们打印到控制台上。
```
sorted_words = sorted(zip(word_len, words))
for length, word in sorted_words:
print(length, word)
```
完整代码:
```
words = input("请输入单词,以空格分隔: ").split()
word_len = [len(word) for word in words]
sorted_words = sorted(zip(word_len, words))
for length, word in sorted_words:
print(length, word)
```
需要注意的是,上述代码中没有考虑到单词长度相同的情况。如果要考虑到单词长度相同的情况,可以将zip()函数替换为一个多维列表,在排序时将第二个值作为辅助排序参数。例如,使用下面的代码:
```
words = input("请输入单词,以空格分隔: ").split()
word_len = [len(word) for word in words]
sorted_words = sorted(zip(word_len, words), key=lambda x: (x[0], x[1]))
for length, word in sorted_words:
print(length, word)
```
这将按照长度排完序之后,如果长度相同,再按照字典排序。
相关推荐
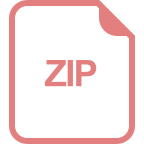
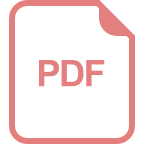
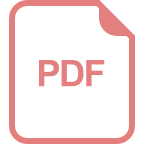














