输入一组字符 统计出其中英文字母 空格 数字 和其他字符个数 用c语言表示
时间: 2024-11-30 21:18:41 浏览: 14
在C语言中,你可以创建一个程序来统计输入字符串中各个类别字符的数量。下面是一个简单的示例,它会接收用户输入的字符串,然后遍历该字符串并计算字母、空格、数字和其他字符的数量:
```c
#include <stdio.h>
#include <ctype.h> // 包含了对字符类型判断的函数
void countCharacters(char *str) {
int alpha_count = 0; // 字母数量
int space_count = 0; // 空格数量
int digit_count = 0; // 数字数量
int other_count = 0; // 其他字符(非字母和数字)数量
while (*str != '\0') { // 遍历直到遇到字符串结束符
if (isalpha(*str)) { // 判断是否为字母
alpha_count++;
} else if (*str == ' ') { // 判断是否为空格
space_count++;
} else if (isdigit(*str)) { // 判断是否为数字
digit_count++;
} else { // 其他字符
other_count++;
}
str++; // 移动指针到下一个字符
}
printf("英文字母: %d\n", alpha_count);
printf("空格: %d\n", space_count);
printf("数字: %d\n", digit_count);
printf("其他字符: %d\n", other_count);
}
int main() {
char input[100];
printf("请输入一个字符串: ");
fgets(input, sizeof(input), stdin); // 获取输入并忽略换行符
input[strcspn(input, "\n")] = '\0'; // 清除fgets带来的换行符
countCharacters(input);
return 0;
}
```
这个程序首先获取用户的输入,然后使用`isalpha()`、`isspace()`和`isdigit()`函数检查每个字符的类型。最后,打印出各类字符的数量。
阅读全文
相关推荐
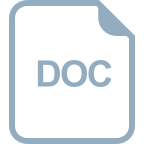
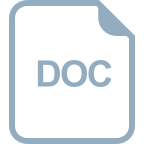
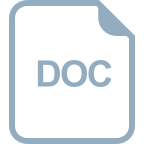















