mixins: [mixin],
时间: 2023-10-31 14:06:35 浏览: 74
Mixins in programming refer to a way of reusing code across multiple classes. A mixin is essentially a class that contains a set of methods and properties that can be added to other classes. When a class includes a mixin, it inherits all the methods and properties defined in the mixin. This allows for code reuse and cleaner, more modular code.
In the code snippet you provided, `[mixin]` is a placeholder for the actual mixin class that you would define. It indicates that the `mixins` property of a class should include the methods and properties defined in the `mixin` class. Here's an example of how you might use mixins in a JavaScript class:
```
class Animal {
constructor(name) {
this.name = name;
}
}
// Define a mixin with some common methods
const swimMixin = {
swim() {
console.log(`${this.name} is swimming`);
},
dive() {
console.log(`${this.name} is diving`);
}
};
// Use the mixin in a class
class Dolphin extends Animal {
constructor(name) {
super(name);
}
// Include the mixin
get mixins() {
return [swimMixin];
}
}
const flipper = new Dolphin('Flipper');
flipper.swim(); // Output: "Flipper is swimming"
flipper.dive(); // Output: "Flipper is diving"
```
In this example, we define an `Animal` class and a `swimMixin` that contains some common methods for swimming. We then define a `Dolphin` class that includes the `swimMixin` using the `mixins` property. Finally, we create an instance of `Dolphin` and call the `swim` and `dive` methods defined in the `swimMixin`.
阅读全文
相关推荐
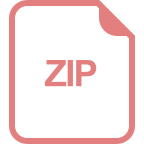
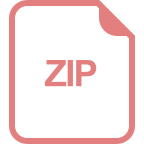
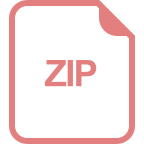


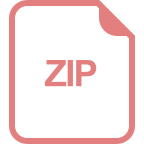
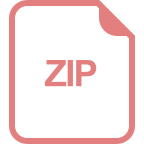
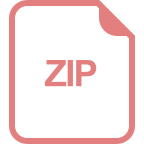
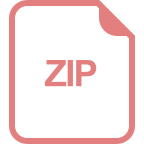
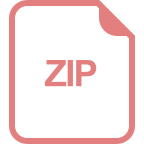
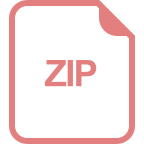
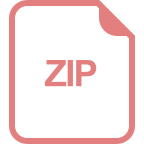
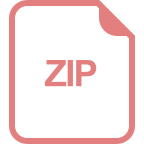
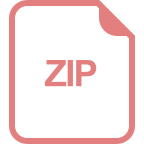
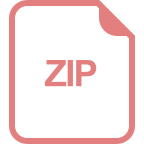
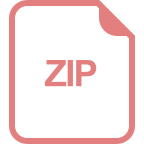


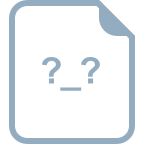