c++:用map。单词查询。能实现模糊查询。如输入字母a,ab,则能展示a或ab为前缀的所有单词。基于树的先序遍历展示。统计每个单词的词频,并能按照字母顺序展示所有单词的词频。
时间: 2024-03-20 13:38:41 浏览: 22
好的,这是一个关于C++中使用map实现单词查询的问题,同时可以进行模糊查询和词频统计。
首先,我们可以使用map来存储单词及其出现的次数,将单词作为key,出现次数作为value。同时,我们可以使用一个set来存储所有的单词,方便后续的展示。
对于模糊查询,我们可以使用map的lower_bound和upper_bound方法,来找到所有符合前缀的单词。具体实现可以参考下面的代码:
```c++
#include <iostream>
#include <map>
#include <set>
#include <algorithm>
using namespace std;
int main() {
map<string, int> freq;
set<string> words;
// 读入单词,统计词频
string word;
while (cin >> word) {
freq[word]++;
words.insert(word);
}
// 展示所有单词及其词频
for (const auto& w : words) {
cout << w << ": " << freq[w] << endl;
}
// 模糊查询
string prefix;
cout << "Enter prefix: ";
cin >> prefix;
auto it1 = freq.lower_bound(prefix);
auto it2 = freq.upper_bound(prefix + char(127)); // char(127)为最大的ASCII码字符
cout << "Words starting with " << prefix << ":" << endl;
for (auto it = it1; it != it2; it++) {
cout << it->first << ": " << it->second << endl;
}
return 0;
}
```
对于按照字母顺序展示所有单词的词频,我们只需要将set中的单词按照字典序排序后,依次输出其词频即可。具体实现可以参考下面的代码:
```c++
// 按照字母顺序展示所有单词的词频
vector<string> sorted_words(words.begin(), words.end());
sort(sorted_words.begin(), sorted_words.end());
cout << "Words sorted by alphabetical order:" << endl;
for (const auto& w : sorted_words) {
cout << w << ": " << freq[w] << endl;
}
```
希望这个代码可以帮助你解决问题!
相关推荐
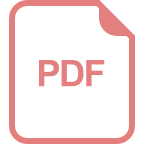
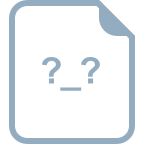














